Creative Coding: An Introduction to Loops in Python with Turtle
Unlock the fun of Python with Turtle graphics! Explore the basics of loops and create captivating patterns with our interactive guide. Visit us for easy-to-follow tutorials that turn coding into a visual adventure.
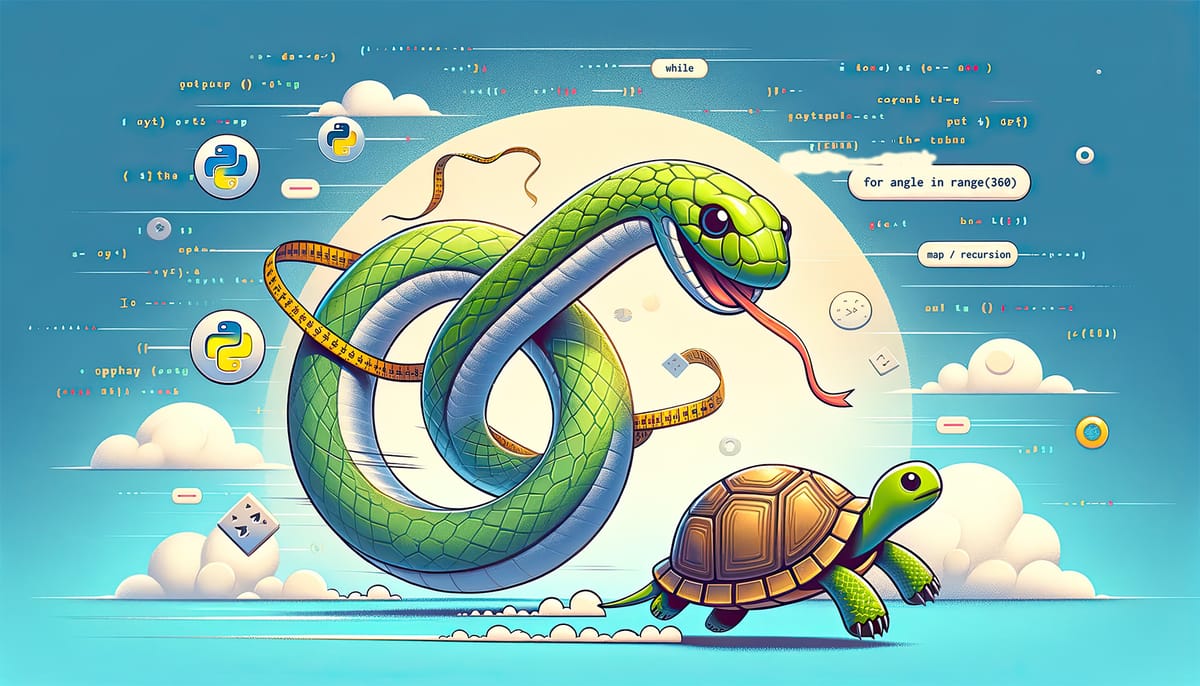
Overview
Hey there, future Python pros! πβ¨
Have you ever thought coding could be as fun as drawing? It can, and this is a throwback since one of my first experiences with computers was using Logo.
Well, prepare to dive into the vibrant world of Python, where we'll turn lines of code into beautiful graphics using loops.
But wait, what's a loop? Imagine repeatedly telling your computer to do something, like drawing a circle or square. That's looping for you β the heart and soul of coding!
Now, let's talk about the Turtle module in Python. No, it's not about slow-moving reptiles; it's a fun tool that enables us to create graphics. Think of it as your digital pen for drawing with code. By the end of this journey, you'll grasp the concept of loops and create some awesome art!
Ready to embark on this coding adventure? Let's get started!
Introduction to Python's Turtle Module
Let's talk about Python's Turtle module, your new best friend in creative coding! π’π¨
First things first, what is this Turtle module? It's a unique Python library that lets you control a turtle (a digital one, of course!) to draw unique patterns and shapes. Think of it as your canvas and paintbrush but in the coding world. It's simple to use, enjoyable, and an excellent way to visualize what your code is doing.
Setting up the Turtle module is a breeze. We're good to go if we've got Python installed.
We need to import the Turtle library at the beginning of our Python script. Once we've done that, we can start commanding your digital turtle.
Letβs do a quick test: ask the turtle to move forward and draw a straight line. Here's how we do it:
import turtle
t = turtle.Turtle()
t.forward(100)
turtle.done()
After writing this, we need to run the script with python turtle_loops.py
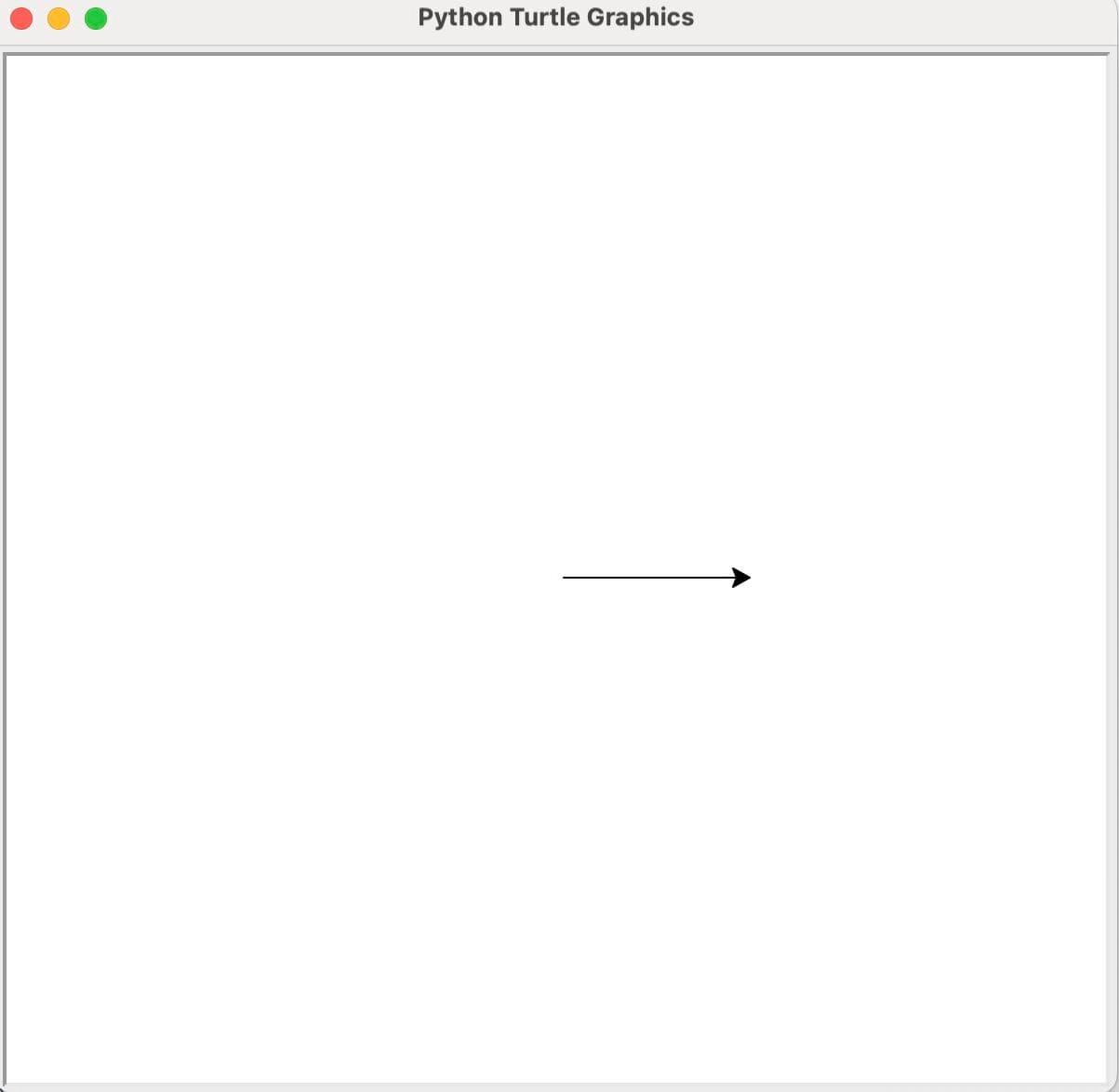
VoilΓ ! You've just made your first turtle move and draw. How cool is that?
Let's see how we can combine Turtle with loops to create genuinely fascinating designs. So, buckle up, and let's get ready to turn lines of code into digital art!
Turtle Turns: A Beginner's Guide to Python Loops
First up, let's talk about loops. In Python, loops are like a superpower for doing repetitive tasks without repeatedly writing the same line of code. We'll focus on two main types of loops: for
loops and while
loops.
For Loops
These are great when you know how often you want to repeat an action. You can tell your turtle to move in a square shape precisely four times, like this:
import turtle
t = turtle.Turtle()
for i in range(4):
t.forward(100)
t.right(90)
turtle.done()
While Loops
These keep going as long as a condition is true. You could tell your turtle to keep moving forward until it reaches a certain point:
import turtle
t = turtle.Turtle()
steps = 0
while steps < 4:
turtle.forward(100)
turtle.right(90)
steps += 1
turtle.done()
Choosing Between For and While Loops
- Use a for loop when:
- The number of iterations is known.
- You're iterating through a collection (like lists, tuples, sets).
- Use a while loop when:
- The number of iterations has yet to be discovered in advance.
- It would be best to keep looping as long as a condition is true.
Combining a for Loop With Turtle
Now, let's combine a for loop with Turtle graphics. Imagine you want to draw a circle made of squares β sounds fun, right? Here's a sneak peek at how you can do it:
import turtle
t = turtle.Turtle()
for i in range(36):
for j in range(4):
t.forward(100)
t.right(90)
t.right(10)
turtle.done()
In this script, the outer for
loop (for i in range(36)
) repeats the drawing of a square 36 times. Each square is drawn by the inner for
loop (for j in range(4)
), turning right 90 degrees after each side to complete the square. After drawing each square, the turtle turns right 10 degrees (t.right(10)
), creating the spiral effect.
The magic number here is 10, the angle by which the turtle turns after drawing each square. Since a complete turn is 360 degrees, turning 10 degrees each time means we'll complete a full circle after 36 squares (360 / 10 = 36). This creates a mesmerizing spiral pattern of squares!
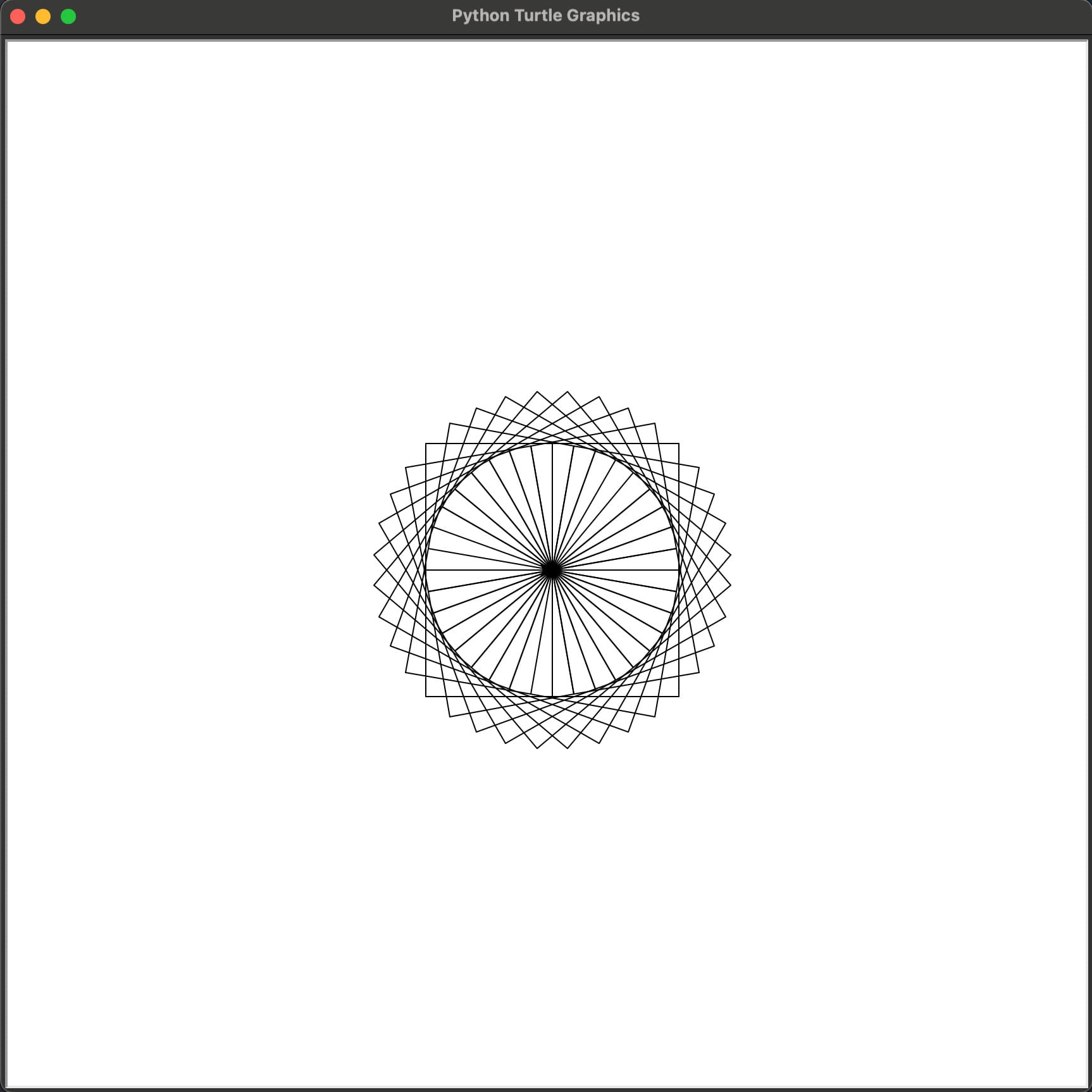
Remember to add turtle.done()
at the end of your script to keep the Turtle graphics window open.
Exciting, isn't it? We've now learned how to create artistic patterns with loops in Python. In the next section, we'll explore more creative applications of loops with Turtle, showcasing the power and fun of programming!
Creating a Compact Spiral with a While Loop
Let's refine our spiral example to create a smaller spiral that stops before leaving the canvas. This will demonstrate how to control the size and boundaries of your drawing with a while
loop:
import turtle
t = turtle.Turtle()
for i in range(36):
for j in range(4):
t.forward(100)
t.right(90)
t.right(10)
turtle.done()
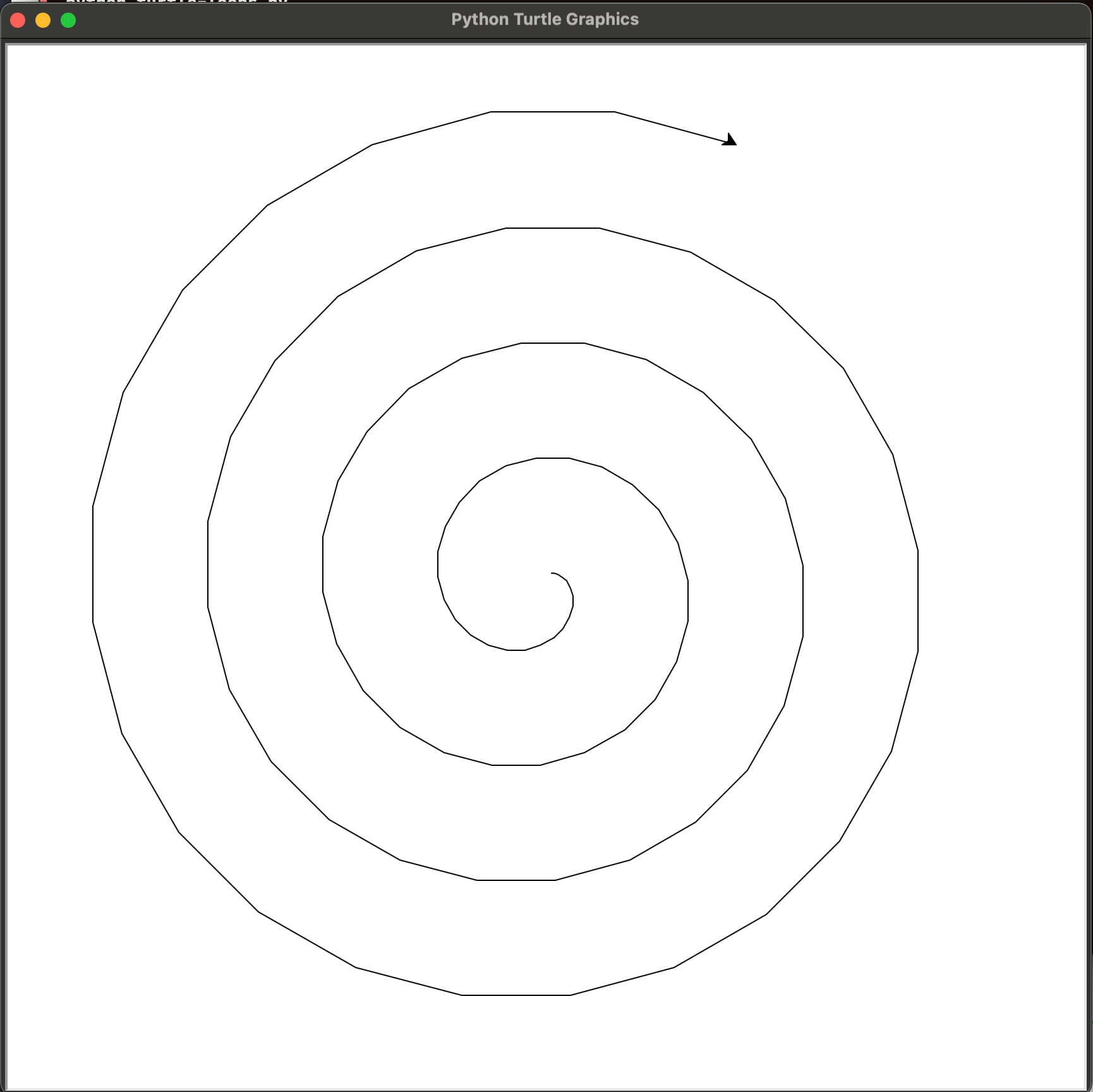
In this script:
- We start with an even smaller distance (
distance = 2
). - The
while
loop condition now includes a maximum distance (distance < max_distance
). - The turtle moves forward by
distance
and turns byangle
(15 degrees) on each iteration. - After each loop,
distance
increases by 1, gradually expanding the spiral.
By setting max_distance = 100
, we ensure the spiral stops growing before it can exceed the size of the Turtle graphics window.
Why This Approach Works
- Controlled Growth: The spiral expands in a controlled manner, stopping before it gets too large.
- Customization: Adjusting the
distance
,angle
, andmax_distance
values allows for different spiral sizes and patterns, showcasing the versatility ofwhile
loops.
Creative Applications of Loops and Turtle
Now that we've got the hang of loops with Turtle let's unleash our creativity! Python's Turtle module and looping constructs can be a powerful tool for creating truly artistic designs. π¨π’
Starry Night with Nested Loops
Have you ever thought of drawing stars in your Python canvas? Nested loops to the rescue! Here's a simple way to create a night sky filled with stars:
import turtle
t = turtle.Turtle()
for i in range(36):
for j in range(4):
t.forward(100)
t.right(90)
t.right(10)
turtle.done()
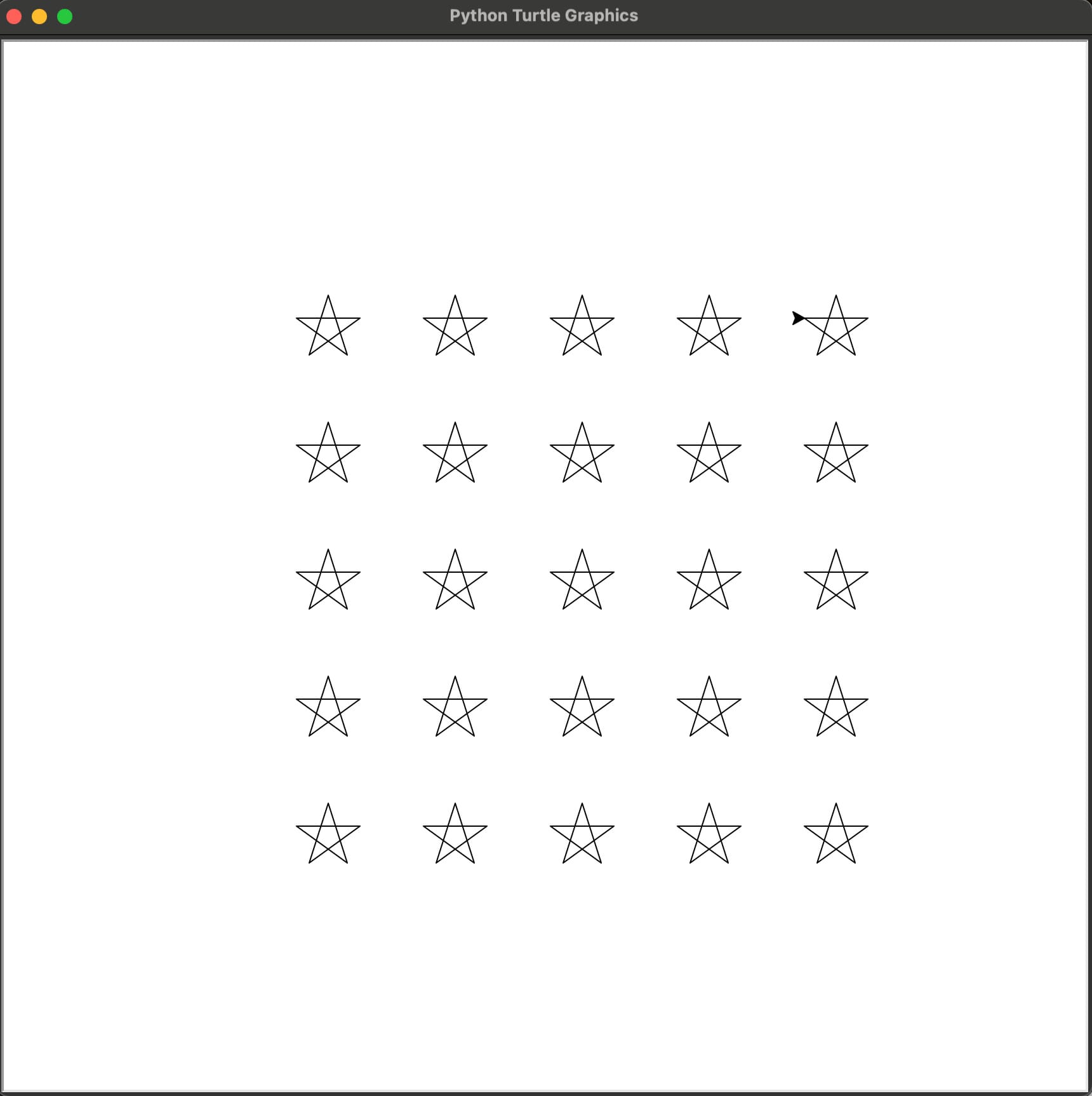
This code creates a grid of stars. The outermost loops (for i in range(5)
, for j in range(5)
) position the turtle for each star, while the innermost loop (for k in range(5)
) draws each star with five points.
Spiraling into Creativity with the While Loop
Letβs take our spiral example a notch higher. We can create an array of mesmerizing designs by slightly altering the parameters in our previous while loop. Here's an example of how to draw a colorful, expanding triangle spiral:
import turtle
t = turtle.Turtle()
for i in range(36):
for j in range(4):
t.forward(100)
t.right(90)
t.right(10)
turtle.done()
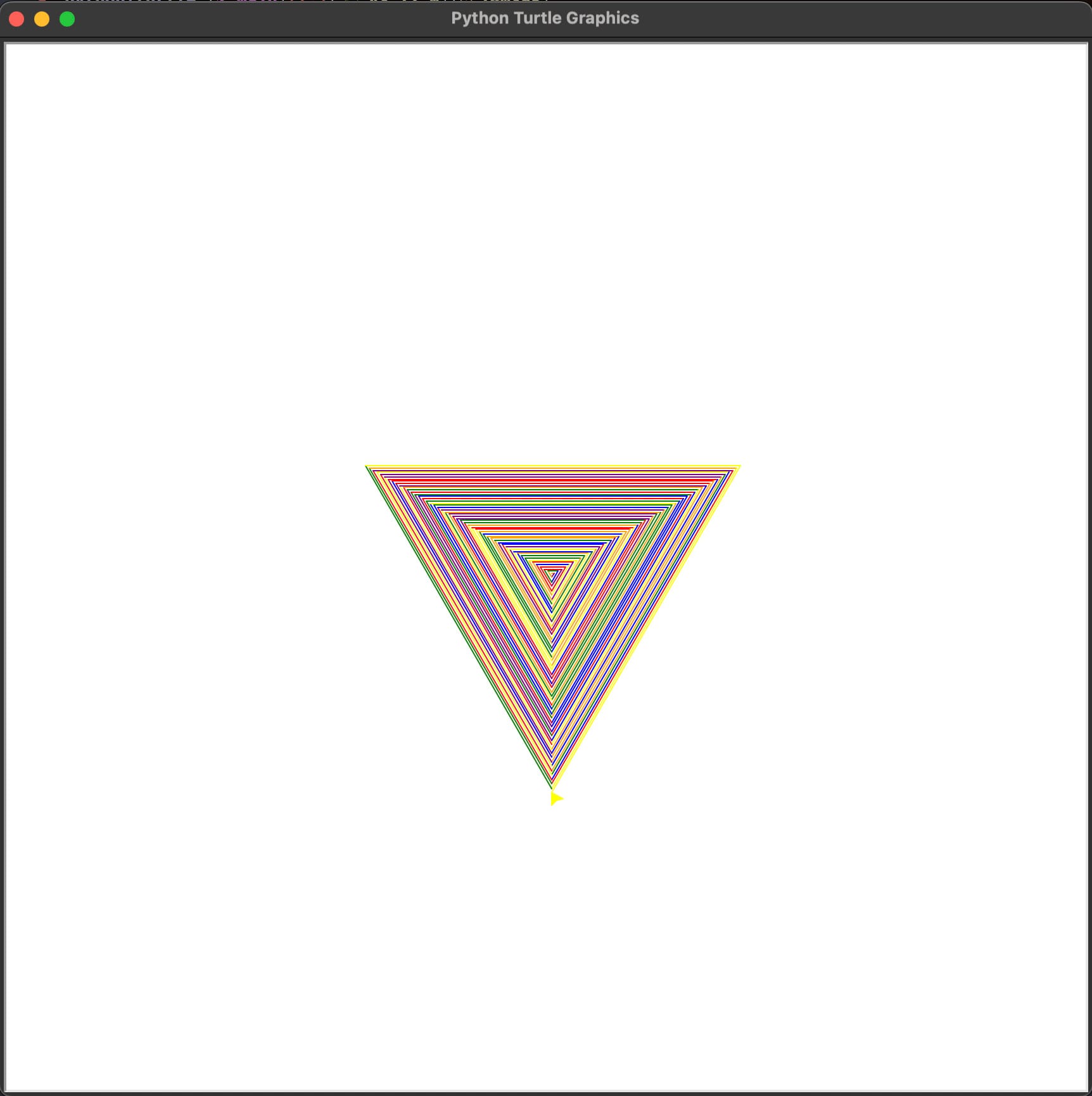
In this script, we introduce random colors from a list, making each spiral segment a different color. The angle
of 120 degrees gives a new twist to our spiral, creating a more complex pattern.
Final Touches
These examples are just the beginning. Experiment with different loop structures, angles, and positions to create unique designs. Remember, coding isn't just about logic; it's also an avenue for artistic expression!
As you explore, remember that mistakes are part of the learning process. If your pattern doesn't turn out as expected, tweak your code and try again. That's the beauty of coding with Python and Turtle: endless possibilities!
π Create Your Turtle Masterpiece! π
It's time to put your new skills to the test! Create a unique piece of art using Python's Turtle module. Unleash your creativity β geometric patterns, abstract designs, or nature-inspired creations are all fantastic!
How to Participate:
- Design something extraordinary using Python and Turtle.
- Capture a screenshot of your artwork.
- Please share it in the comments below or on social media. Use the hashtag #TurtleArtChallenge and tag us on Instagram @The_Turing_Taco_Tales.
Let's fill the digital world with your incredible Turtle art! Can't wait to see what you create.
Happy coding, and let your imagination soar!
Conclusion
And that's a wrap on our exciting exploration of loops and Turtle graphics in Python! π Remember, each line of code is a step closer to becoming a programming whiz.
Keep experimenting, creating, and enjoying the journey. For more coding adventures, subscribe to TuringTaco.com. Share your Turtle creations using #TurtleArtChallenge and tagging @The_Turing_Taco_Tales on Instagram.
Until next time, happy coding! π©βπ»π¨βπ»
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 β¬ on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
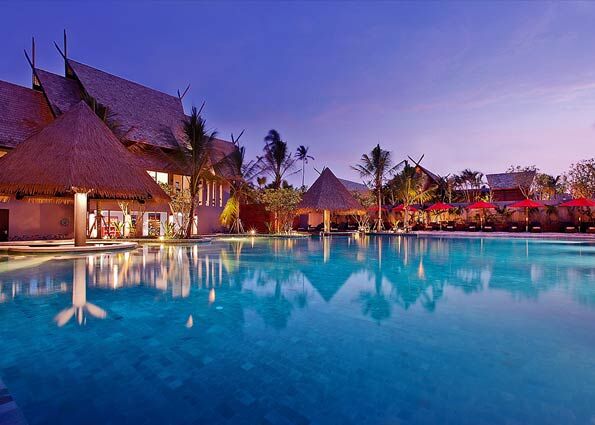
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: