Exploring Literal Types for Robust Python Programming
Discover the power of Python 3 Literal Types in our detailed article. Learn about their role in enhancing code clarity, enabling precise static type checking, and optimizing API design.
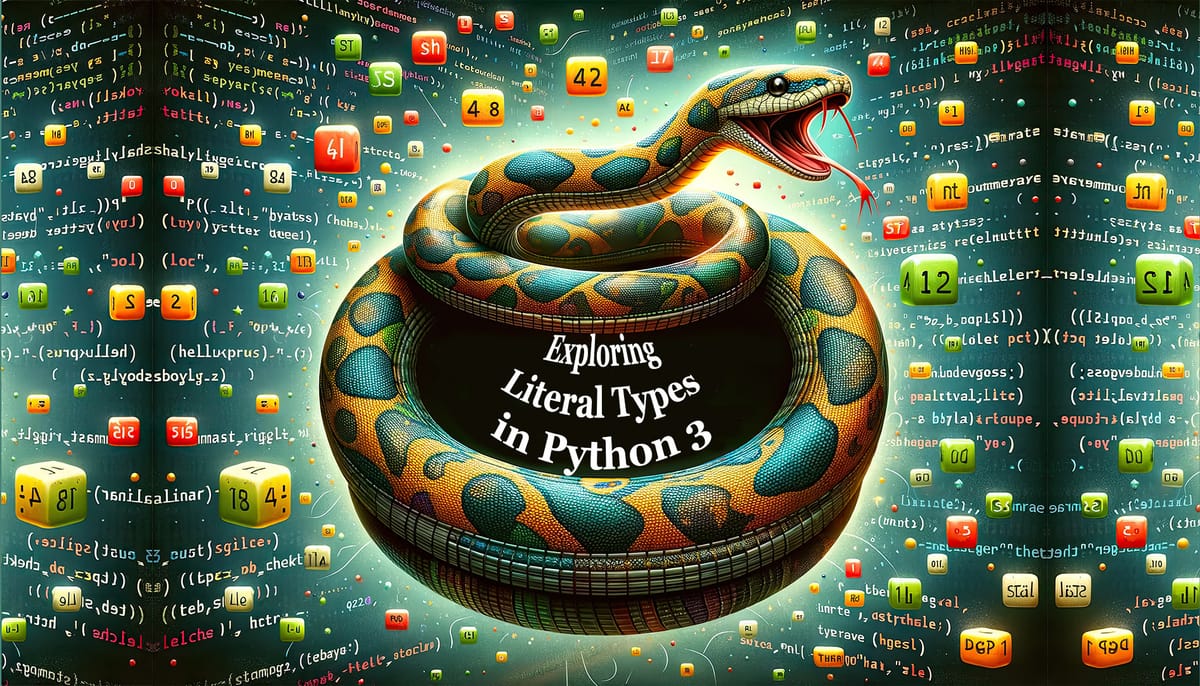
Overview
Hey there, fellow Python enthusiasts! We've all seen how type hints have been a game-changer, making our Python code easier to understand and more manageable. Our previous article, "Type Hints: Python’s Weapon Against Code Anarchy," discussed optional type hints. In this article, we're diving further into Python 3's typing by looking at Literal Types.
Literal Types enhance Python type-checking capabilities, allowing us to differentiate between values written directly in our programs and values from outside the program as input; for this distinction, the source of the input data is not essential.
Whether just getting started with Python or a seasoned pro looking for new tricks, understanding Literal Types is a great way to sharpen your coding skills.
What are Python 3 Literal Types?
Literal Types were introduced in Python 3.8; they increase the utility of type hinting by allowing us to define the exact values a variable can hold. These values are fixed literals, written directly into the code rather than being calculated or input during runtime.
Let's consider a practical example involving a SQLite database to illustrate how Literal Types can be effectively used:
import sqlite3
from typing import Literal, List
# Create a SQLite database and a 'users' table
conn = sqlite3.connect(':memory:')
# Using an in-memory database for simplicity
cursor = conn.cursor()
cursor.execute("CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT, email TEXT)")
cursor.execute("INSERT INTO users (name, email) VALUES ('Alice', 'alice@example.com')")
cursor.execute("INSERT INTO users (name, email) VALUES ('Bob', 'bob@example.com')")
conn.commit()
# Function to query the users table
def query_users(column: Literal['id', 'name', 'email']) -> List[tuple]:
cursor.execute(f"SELECT {column} FROM users")
return cursor.fetchall()
# Usage of the function
print(query_users('name')) # Valid usage
print(query_users('email')) # Valid usage
# The following should be caught as an error
# by a type checker like mypy
print(query_users('password'))
# Invalid column, not part of the literal type
In this example, the function query_users
uses Literal Types to specify which columns can be queried. The argument column
is restricted to the literals 'id,' 'name,' or 'email,' directly reflecting the columns in the database. This usage of Literal Types provides a clear, error-resistant way to interact with the database. If an invalid column, like 'password', is used, static type checkers such as Mypy will flag this as an error, preventing potential bugs at runtime.

mypy
showing an error when passing an unexpected valueThis example showcases how Literal Types enhance code clarity and enforce database integrity by restricting function parameters to specific, permissible values.
To get the maximum benefit from using Type Literals, we should use a type checker that has already implemented support for Literal Types, like Mypy or Pyre.
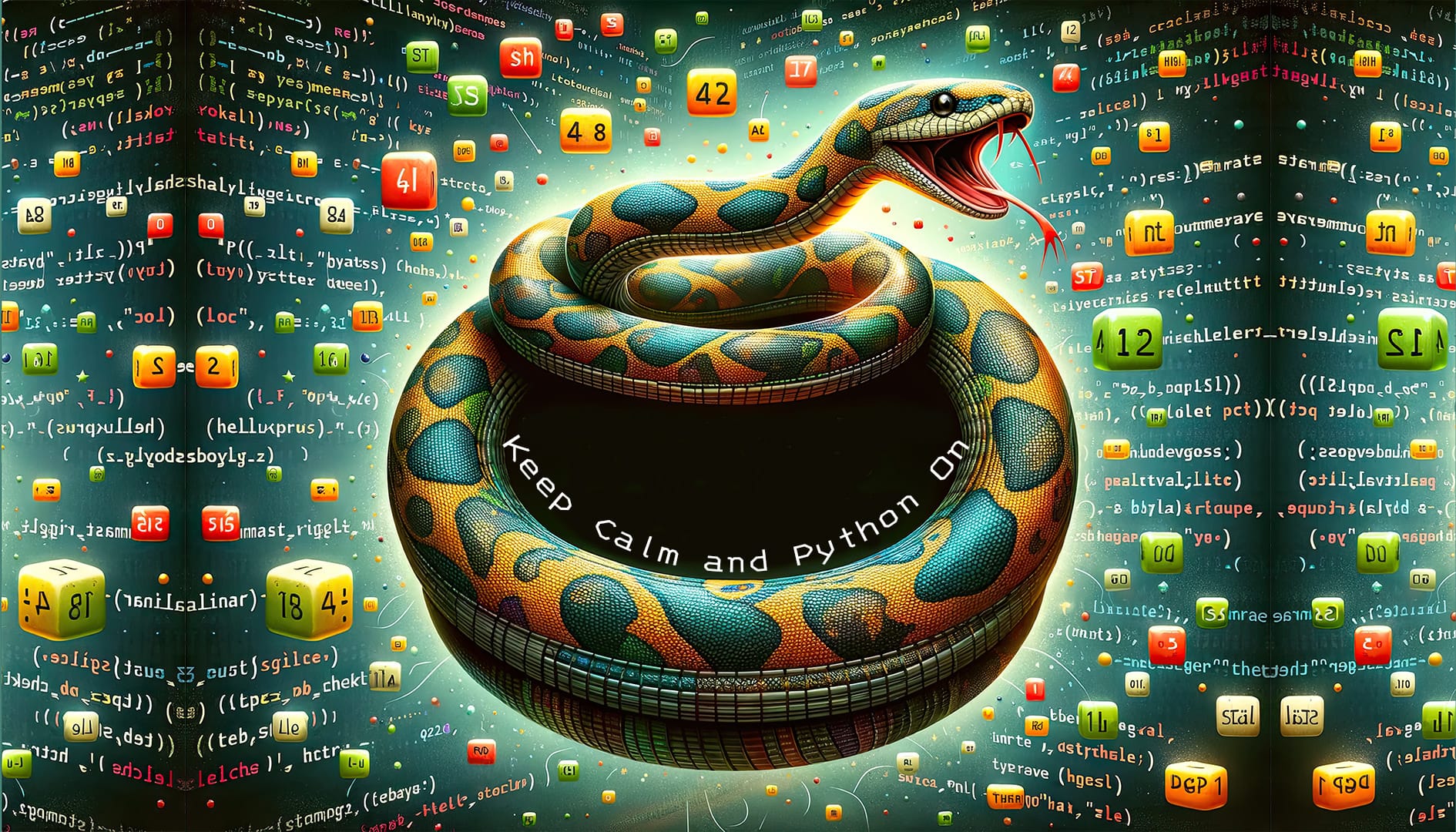
The Rules of Python 3 Literal Types
PEP 586 defines the guidelines that shape how we can use Literal Tyes in our programs:
Core Rules:
- Literal Types can be specific instances of basic types such as
int
,str
, bool, andenum.Enum
- Equality among Literal Types is determined by both type and value. For example,
Literal[3]
is equal to anotherLiteral[3]
, but it differs fromLiteral[4]
orLiteral['3']
.
Literal Types Nesting and Invariance:
- When Literals are nested within each other, Python flattens them. So,
Literal[Literal[3]]
simplifies toLiteral[3]
. - If Literals are combined with Union, Python merges them into a single Literal. For instance,
Union[Literal[3], Literal[4]]
simplifies toLiteral[3, 4]
. - When combined with
Optional
, Literals are unified into one. Hence,Optional[Literal[3]]
is effectivelyLiteral[3, None]
. - Literal Types are invariant, meaning a Literal of a subtype is not a subtype of the Literal of its supertype. For example, though
int
is a subtype ofobject
,Literal[3]
is not a subtype ofLiteral[object]
.
Parameters That Are Not Allowed:
- Non-Literal Values: Literal Types cannot include values that are not literal. This means variables or expressions evaluated at runtime are not permissible as Literal Types.
- Unsupported Types: As mentioned earlier, only specific types (
int
,str
,bool
, andenum.Enum
) are allowed. Using unsupported types likefloat
,complex
, or custom classes as Literal Types will lead to errors.

mypy
showing the error when using a float as a type parameter to Literal- Dynamic Values: Literal Types are meant for static, unchanging values. Parameters involving dynamic computation or changing over time are unsuitable for Literal Types.

mypy
showing the error when using an expression as a type parameter to LiteralUnderstanding and applying these rules empowers us to fully leverage Literal Types' potential in Python. It ensures that your code is precise, highly readable, and maintainable, with fewer errors and misunderstandings.
The Utility of Python 3 Literal Types
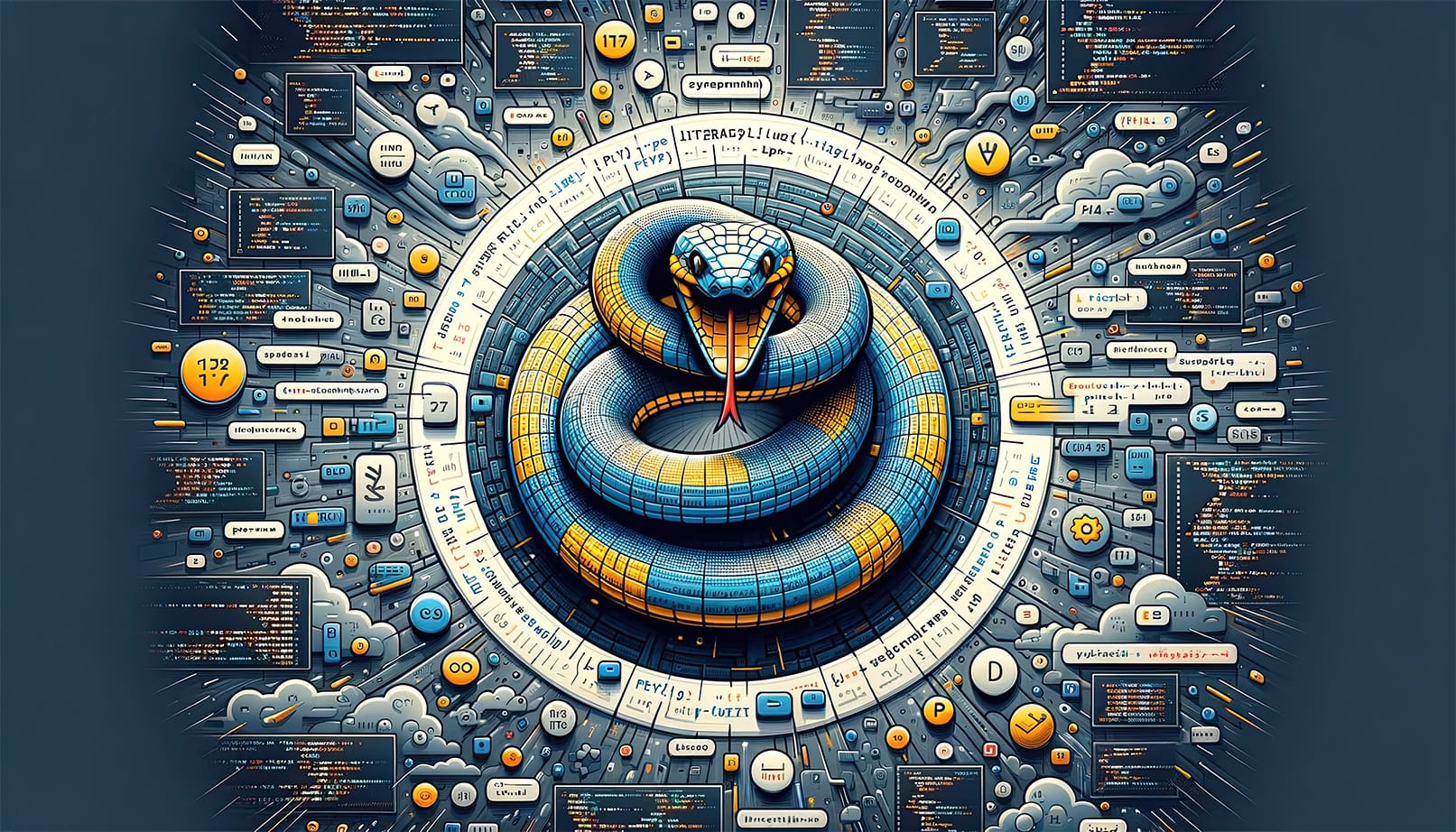
Introducing Literal Types in Python 3.8 opened up new avenues for developers to write more precise and easily understandable code. Their utility in various programming scenarios is significant, enhancing both the functionality and the clarity of code. Let's delve into some of the key areas where Literal Types prove to be especially useful.
Enhanced Code Clarity and Safety:
- By specifying exact values that variables or function parameters can hold, Literal Types make the intentions of your code crystal clear. This specificity reduces ambiguity, making the code easier to read and understand.
- Literal Types also add an extra layer of safety. They enable static type checkers to catch incorrect usage of function arguments or variable assignments, which can prevent bugs that might not be obvious at first glance.
Improving API Design:
- Clarity and correctness are paramount in the design of APIs, especially those that are public and used by a wide range of clients. Literal Types can specify precisely what values an API can accept or return, reducing the likelihood of incorrect usage.
- For instance, if your API has a function that accepts a specific set of commands as strings, using Literal Types can explicitly state which commands are valid, making the API more user-friendly and robust.
Facilitating Better Code Autocompletion:
- Modern IDEs and code editors often rely on type hints to provide intelligent autocompletion suggestions. When Literal Types are used, these tools can offer more accurate suggestions based on the specific values allowed, enhancing the developer experience.
- This leads to faster coding, as developers spend less time remembering or looking up the exact values required by a function or a class.
Useful in Configuration and Settings:
- In settings or configuration files where only a specific set of values is valid, Literal Types can enforce these constraints directly in the code. This makes the configuration more robust and less prone to errors.
- For example, a configuration setting that determines the mode of an application (like 'test', 'development', or 'production') can be strictly enforced using Literal Types.
Optimization in Conditional Logic:
- Literal Types can make the code more predictable and optimized in scenarios involving conditional logic based on specific values. Since the range of values is known and limited, compilers and interpreters can optimize the execution paths more effectively.
- This can improve performance, especially in critical code sections where efficiency is vital.
In summary, Literal Types in Python provide a valuable tool for enhancing code quality across various dimensions - clarity, safety, API design, developer experience, and performance. Their introduction reflects Python's ongoing commitment to improving both the efficiency and readability of code.
Conclusion
As we wrap up our exploration of Python 3 Literal Types, it's evident that this feature is a significant leap forward in Python's journey towards enhancing code clarity and precision. Literal Types provide a way to write more explicit and error-resistant code and reflect Python's adaptive nature, continuously evolving to meet the needs of its diverse user base.
We encourage you to experiment with Literal Types in your projects and see the benefits for yourself.
If you've found this exploration insightful, consider subscribing to The Turing Taco Tales for more enlightening content on Python and other programming topics. Stay ahead of the curve in the ever-evolving world of technology, and let's continue to grow and learn together in this exciting coding journey!
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
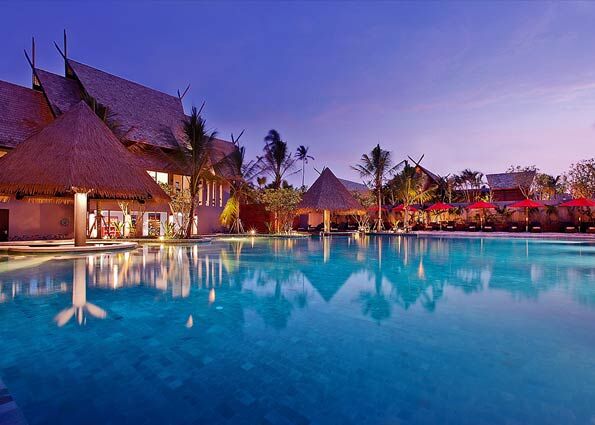
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: