Functors: The Spice of Functional Code
Delve into the basics of Functors in functional programming, exploring their definition, laws, and benefits. Discover through Scala 3 examples of how Functors lead to code reusability and set the stage for advanced functional concepts like Applicatives and Monads.
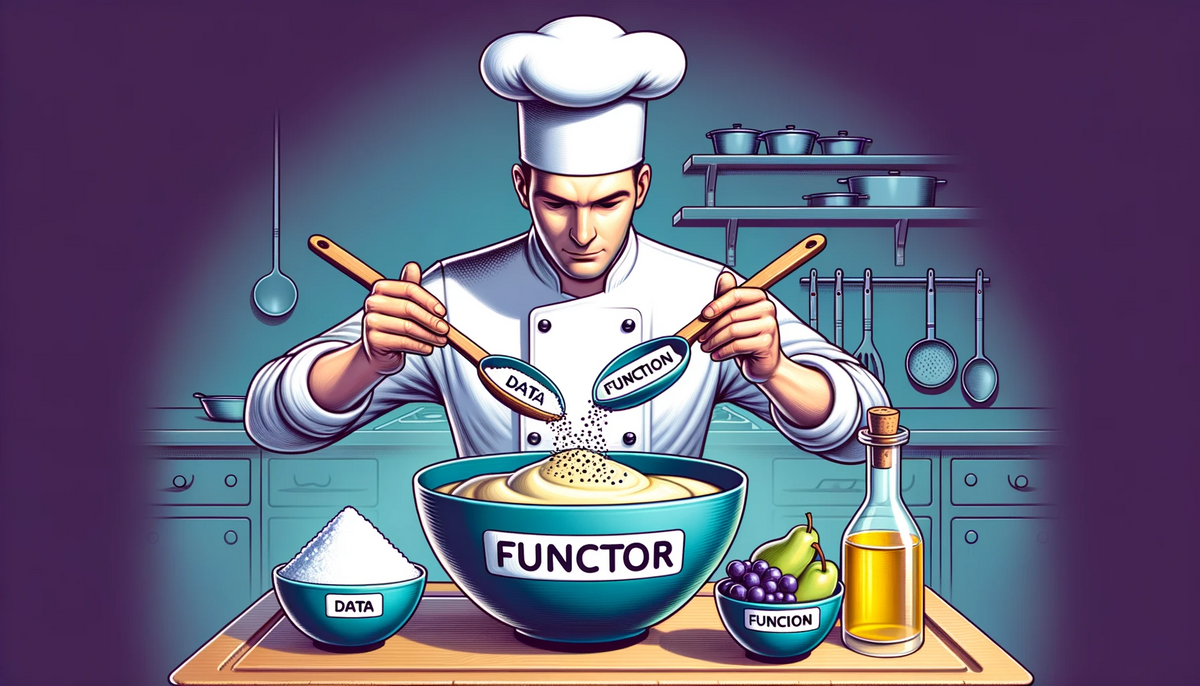
Overview
In our last dive into Algebraic Data Types (ADTs), we explored Sum and Product types. They're great for structuring data, but how do we interact with this data in a type-safe way?
That's where Functors come in. A Functor is a particular type of ADT that lets us apply functions to the data it holds without extracting it. Not all ADTs are Functors, but when an ADT implements some functions and follows specific rules, it becomes a Functor.
In this article, we'll dig into Functors and see how they let us work with data in ADTs. We'll review how to write simple Functors in Scala 3, diving into the map function at the heart of Functors. Let's dive into the world of Functors and see how they add a layer of abstraction and reusability to our code, making our journey into functional programming more exciting and insightful.
Functors and Their Laws
What Is a Functor?
A Functor is a design pattern that arises naturally in many programming contexts. In Scala, we represent Functors as a trait our data types can implement. The trait contains a single method named map.
trait Functor[F[_]] {
def map[A, B](fa: F[A])(f: A => B): F[B]
}
In this trait definition:
- F is a type constructor that takes one type argument (it's a generic type). The underscore F[_] signifies a type constructor.
- map is a method that takes two arguments:
- fa is the functor instance carrying values of type A. It has the type F[A]
- f is a function that transforms an A into a B.
The result of map
is a new functor instance carrying values of type B. Hence, the resulting Functor type is F[B].
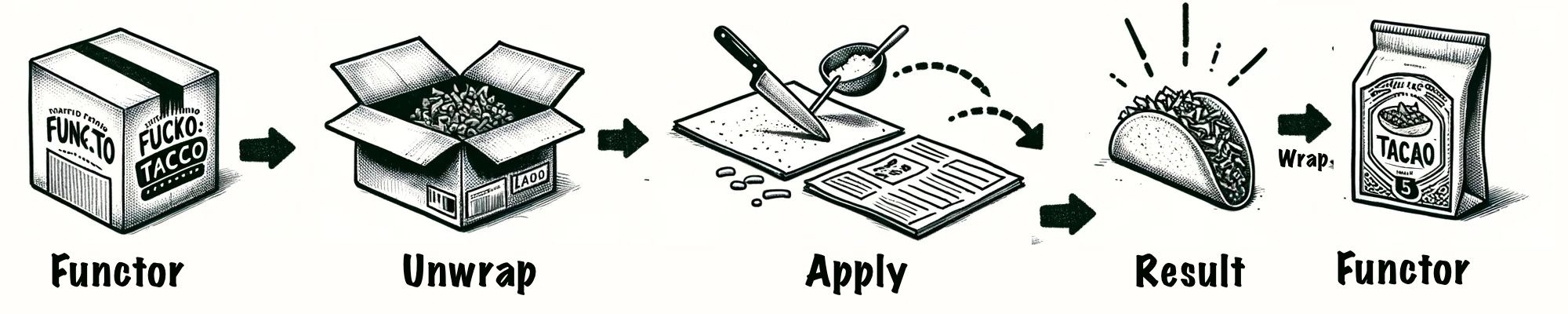
Functor Laws
For a data type to be a proper functor, it must satisfy two laws that ensure the map function behaves in a consistent, predictable manner.
-
Identity Law: Mapping the identity function over a functor should result in precisely the exact functor.
-
Composition Law: Mapping two functions in sequence is the same as mapping their composition.
These laws ensure that the map method is well-behaved and interacts nicely with other operations. They provide a foundation that allows us to reason about the behavior of our code, making our programs more straightforward to understand and refactor.
In the next section, we'll illustrate the Functor trait and its laws with concrete examples in Scala.
Benefits of Functors
Code Reusability
Functors encapsulate a typical pattern of data transformation. We can write generic, reusable code that works across various data types by abstracting this pattern into an interface.
This reusability reduces code duplication, making our codebase cleaner and easier to maintain.
Composability
Functors are composable, meaning we can chain operations together cleanly and readable. The ability to transform data within a context without altering it is a powerful feature that promotes composability.
// Chaining operations using map
val result = List(1, 2, 3).map(_ + 1).map(_ * 2)
// result: List(4, 6, 8)
Predictability
The Functor laws provide a level of predictability. As long as the Functors we use adhere to the identity and composition laws, we can write generic code that will work with different Functors. And we can be sure the code will not only execute but execute correctly regardless of the specific functor implementation.
This predictability makes it easier to reason about our code and understand its behavior.
// Demonstrating identity law with a List Functor
assert(List(1, 2, 3).map(identity) == List(1, 2, 3))
Better Abstraction
Functors abstract the details of transforming data, allowing developers to focus on higher-level logic. This abstraction makes our code more readable and easier to understand, facilitating better collaboration among developers.
We can use Functors to write cleaner, more abstract, and more reliable code.
A Functor in Action: Reusability Across Data Types
The essence of functional programming lies in building reusable, composable abstractions. Functors embody this principle by providing a uniform interface to map over a structure without altering it. Let's illustrate this with a simple example in Scala 3.
We'll implement a simple Functor trait and its fmap
method in our example. We'll then create instances of this trait for some common Scala data types: List
and Option
.
This will allow us to use the same map
function to transform the contents of both a List
and an Option
, demonstrating the power and reusability of Functors.
Let's define our Functor
trait:
// Defining Functor trait with fmap method to avoid name clash with built-in map method in some collections
trait Functor[F[_]] {
def fmap[A, B](fa: F[A])(f: A => B): F[B]
}
// Implementing Functor for List
given Functor[List] with
def fmap[A, B](fa: List[A])(f: A => B): List[B] =
fa.foldRight(List.empty[B])((item, acc) => f(item) :: acc)
// Implementing Functor for Option
given Functor[Option] with
def fmap[A, B](fa: Option[A])(f: A => B): Option[B] =
fa match {
case Some(value) => Some(f(value))
case None => None
}
// Example usage
val listFunctor = summon[Functor[List]]
val optionFunctor = summon[Functor[Option]]
val numbers = List(1, 2, 3, 4, 5)
val maybeNumber = Option(5)
// Using fmap to apply a function to the contents of a List
val incrementedNumbers = listFunctor.fmap(numbers)(_ + 1)
// Using fmap to apply a function to the contents of an Option
val incrementedMaybeNumber = optionFunctor.fmap(maybeNumber)(_ + 1)
In this example, we defined a Functor trait and used map
as the name for the method to avoid name clashes with Scala's built-in methods. We then provided implementations of Functor for List and Option. These implementations define how to apply a function to the contents of a List or Option without mutating the structure itself.
The fmap
implementation doesn't rely on pre-existing map methods. This way, the example shows how to implement it on our types.
Finally, we implemented the increment function that takes a functor instead of a concrete type. And showcase the reusability by applying the function to objects of different types.
This concept is one of the many exciting features of Scala 3, like Higher Kinded Polymorpysm, which we'll explore in depth in the upcoming Discovering Scala 3 series.
Conclusion
We've traversed the fundamentals of Functors, delving into their definition, laws, and benefits, and even got our hands dirty with some Scala code.
The journey enlightened us on how Functors provide a structured way to handle function applications inside a context, a cornerstone of functional programming.
This exploration is a stepping stone towards grasping more complex abstractions in functional programming. As we've seen, Functors are simple, yet they lay the groundwork for more advanced concepts like Applicatives and Monads, which we'll explore in the upcoming articles.
As we wrap up, the invitation is open to dive deeper, explore further, and tune in for the upcoming adventures in the functional programming landscape. Our next stop in this series will venture into the land of Applicatives. Until then, happy coding!
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
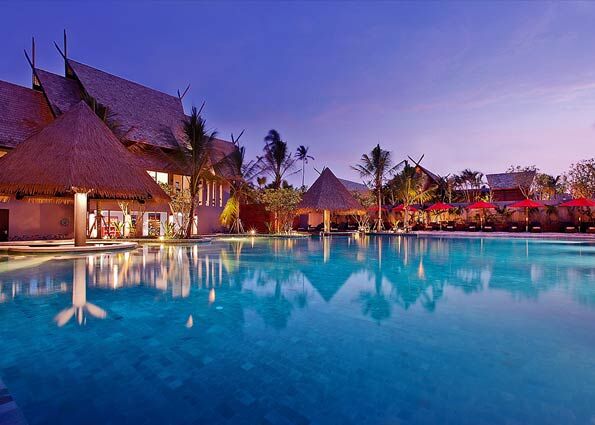
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: