Quick Review of Python's Comprehensions
This tutorial explores Python comprehensions. Learn how to simplify your code and make it more Pythonic by using comprehensions to build dictionaries, sets, and lists. deal for both novices seeking to learn and seasoned programmers looking for a quick refresher.
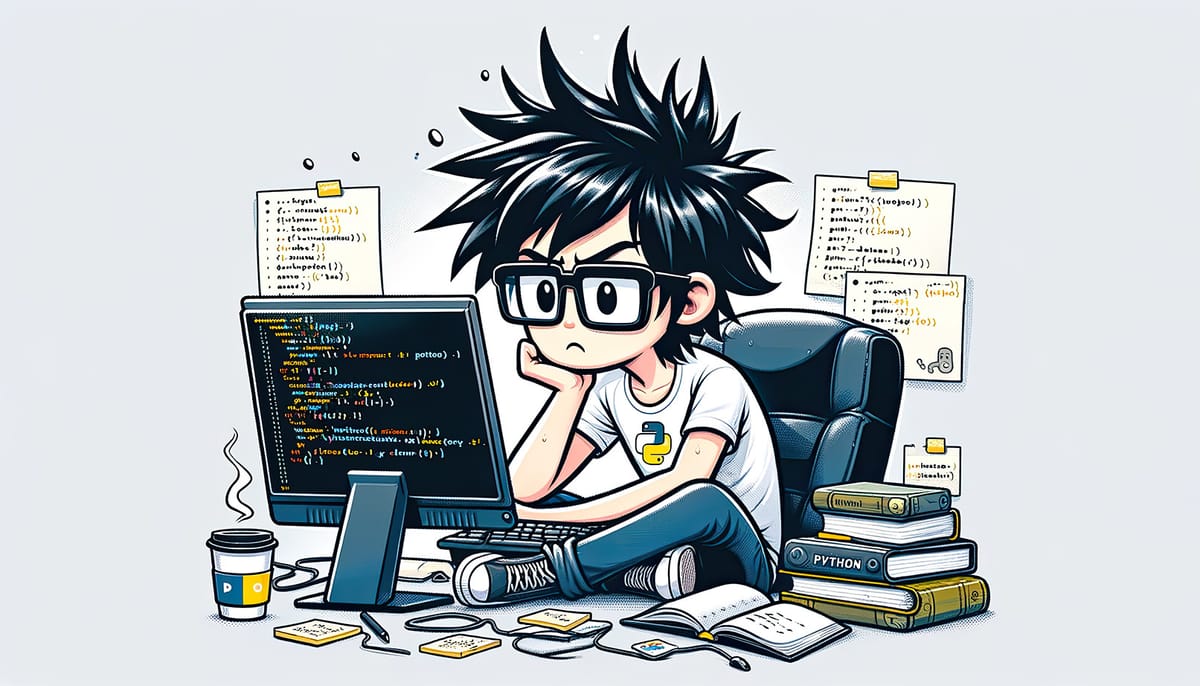
Overview
In mathematics, we can use Set Builder Notation to declare sets based on other sets by defining the rules an object has to meet to be considered an element of the set.
With this notation, we declare a new set S with the following notation { f(x) ∣ x ∈ D, P(x)}
where:
- x is an element from the domain D.
- D is the domain or the original we are using as a basis to derive our new set
- f(x) is a function applied to the elements of the original set to transform them into elements of the new set
- and P(x) is a property or condition that objects of the set must satisfy for f(x) to be part of the new set.
Python adopted this concept early, in the form of list comprehensions, and later extended it to sets and dictionaries.
Python comprehensions are a form of declarative programming, enabling us to declare what rules the collection members should follow instead of exhaustively enumerating all elements.
They are a beautiful example of how adopting mathematical concepts in programming leads to code that is concise and easier to write and maintain.
List Comprehensions
Python's syntax for list comprehensions is similar to the set builder notation. But uses square brackets instead of curly braces and the keywords for/in to define the universe and if
to introduce the predicate:
[expression for item in iterable if condition]
The expression is any valid Python expression that will be evaluated on each item.
The predicate is optional; if it is not included, all the results of applying the expression to the original collection items will be included in the resulting list. If included, the predicate will be evaluated, and the value produced by the expression will be part of the resulting list when the predicate is valid.
# Squares from 1 to 5
squares =[x**2 for x in range(1, 6)]
# Even squares from 1 to 5
even_squares = [num**2 for num in range(1, 6) if num % 2 == 0]
Comprehensions are more concise, readable, and often better performing than traditional loops to create a list.
Set Comprehensions
Python also supports set comprehensions, which we can use to create collections without repeated elements based on other collections. Syntactically, the only difference in list comprehensions is that we write them using curly braces instead of square brackets:
# Unique square numbers
unique_squares = {x**2 for x in range(-4, 5)}
# Get unique occurrences from a list using a set comprehension
unique_even = {x for x in [1, 1, 2, 2, 3, 3, 4, 4] if x % 2 == 0 }
Dictionary Comprehensions
Since the inclusion of PEP-274 in Python 2.7 and 3.0, we can create new dictionaries from other tables using comprehension syntax. The syntax changes are minimal. We write them using curly braces like set comprehensions but add an expression defining the key for the dictionary and separate it from the expression defining the value using a colon:
{key_expression: value_expression for item in iterable if condition}
In action, they look as concise as the previous comprehensions; for example, we can create a dictionary of numbers and their squares in a single line:
squares_dict = {num: num**2 for num in range(-4, 6)}
Dictionaries ensure the uniqueness of the keys. Hence, we'll have repeated values instead of the set comprehension example in the above dictionary.
Conclusion
We can use Python's comprehensions to create new collections in an idiomatic way.
Like with any other feature, we should prioritize readability and maintainability. We should avoid inlining overly complex expressions and use functions with descriptive names.
Besides, using the right kind of comprehension will help us avoid unnecessarily creating intermediate collections, preventing performance problems in our code.
We hope you have found this quick comprehension helpful review; we would appreciate it if you subscribe and comment about comprehension or suggest other topics you would like us to write about.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions, you can read the full story here.
In the meantime, I decided to accept donations.
If you can afford it, please consider donating:
Every donation helps me offset the running costs of the site and an unexpected tax bill. Any amount is greatly appreciated.
Also, if you are looking to buy some Swag, please visit I invite you to visit the TuringTacoTales Store on Redbubble.
Take a look, maybe you can find something you like: