Monad Mixology: Harnessing Composability for Better Code
Delve into Monads, key components in functional programming, through clear definitions, laws, and a practical example. Uncover their ability to handle program-wide concerns like side effects, paving the way for mastering advanced functional programming concepts.
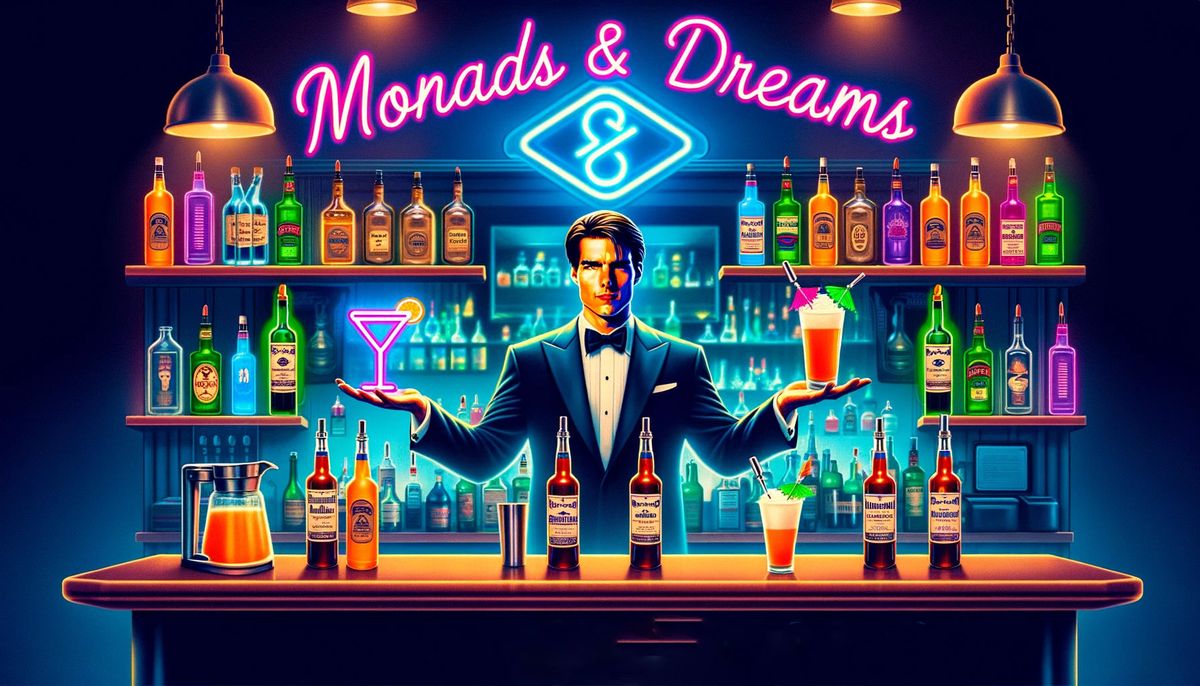
Overview
Monads are a big step in functional programming. They help us manage side effects in code, making it clean and type-safe. In earlier articles, we explored Functors and Applicatives. Now, we move to a more profound concept - Monads. They are often called "programmable semicolons" due to their ability to manage operations in sequence.
Monads have a rich structure. They help our code flow smoothly, especially when dealing with specific effects like optionality, state, or IO. They are like a well-planned railway system, moving data from one computation to the next, even when there are bumps or crossroads.
In this article, we'll explore the core of Monads, their laws, and how they handle side effects. This makes our code more readable and maintainable. Through practical examples, we'll understand Monads better. They make our code easy to write and maintain, blending well with functional programming principles. So, let's dive into the world of Monads and see how they can improve our code.
Defining Monads: Structure and Laws
Monads are a fundamental concept in functional programming. They are a progression of the theoretical concepts we explored in previous articles (ADTs, Functors & Applicatives). They have a special place in programming due to their ability to handle program-wide concerns, such as state or I/O, in a purely functional programming way.
A Monad design pattern handles program-wide concerns, such as state or I/O, within pure functional programming. At its core, a Monad is defined by two operations: bind
(or flatMap
) and unit
(or pure
).
Every Monad is also an Applicative Functor. This relationship is fundamental and showcases how these structures build upon one another.
The Interface of a Monad
To be a monad, an ADT should provide the following methods: pure
and bind
.
unit
(or pure
) is the same as the pure
method we saw in Applicatives, but it lifts the value into a monadic container instead of an Applicative one.
bind
(or flatMap
) is the operation that makes Monads genuinely shine. It takes a monadic value and a function that takes a plain value and returns a monadic value. It applies the function and returns the monadic result directly without wrapping it in another context.
If we were to map a function A => F[B]
over Functor, we would end up with a nested value F[F[B]].
The process of eliminating multiple nesting contexts and leaving only one is often known as flattening, and this is the reason why bind
is named flatMap
in some languages. It could be understood as mapping and then flattening the result.
Monad Laws
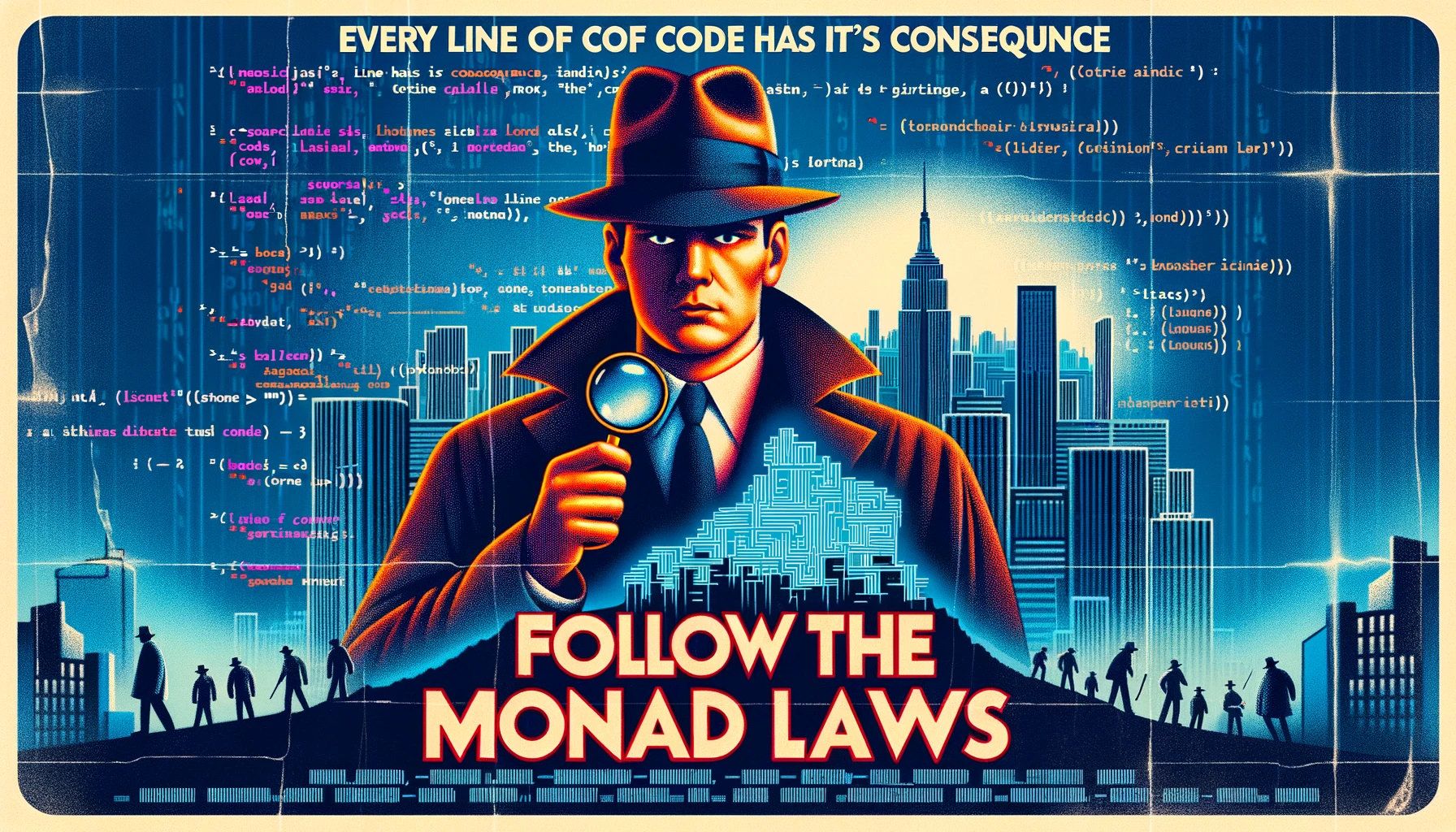
There are three fundamental laws that Monads must satisfy to ensure consistent behavior across different implementations. These laws help us reason about the behavior of monadic operations and create a predictable framework for working with Monads.
While defining the laws, we will refer to the operations as unit
to differentiate them from the Applicative pure
and bind
to because it is shorter than flatMap
Left Identity bind(unit(x), f) == f(x)
The left identity law ensures that if you take a value, put it in a default context with unit
, and then feed it to a function via bind
, it's the same as just taking the value and applying the function to it directly.
Right Identity bind(Mx, unit) == Mx
The right identity law ensures that if we have a monadic value and use bind to feed it to the unit
, we get the same monadic value back.
Associativity flatMap(flatMap(mx, f), g) == flatMap(mx, x => flatMap(f(x), g))
The associativity law ensures that when we have a chain of monadic function applications, it doesn't matter how we nest them; the result will be the same.
Link to Applicative Functor
The operations of a Monad align with those of an Applicative Functor, with a twist. While an Applicative Functor's apply
method allows us to use a function wrapped in a context to a value wrapped in a context, a Monad’s flatMap
method takes this further. It will enable us to apply a function that returns a wrapped value to a wrapped value and flattens the result into a single wrapped value.
In essence, while Applicative Functors allow us to apply functions to values in a context, Monads enable us to chain such operations, effectively dealing with nested contexts. This added capability of Monads to handle the nesting of contexts makes them more powerful and expressive.
Furthermore, you can derive the apply
and map
methods of an Applicative Functor from the flatMap
and pure
methods of a Monad. Here's how you could do it:
def apply[A, B](mf: M[A => B])(ma: M[A]): M[B] = flatMap(mf, f => map(ma, f))
def fmap[A, B](ma: M[A], f: A => B): M[B] = flatMap(ma, a => pure(f(a)))
As long as the Monad we start with obeys the Mondad laws, we can be sure the derived Applicative and Functor instances will follow their respective laws.
This connection shows the elegant hierarchy and the progressive enhancement of capabilities from Functors to Applicatives to Monads, each layer adding more power to handle complex operations and manage side effects in a composable and type-safe manner.
Showcasing Monads with a Parsing Example
Monads shine in many practical scenarios, and parsing is one of them. Let's create a simple parser that reads integers and characters from a string.
We aim to chain parsers together to process a string sequentially, demonstrating how monads facilitate such compositions.
Defining the Monad and Parser
We'll define a generic Monad
trait and a specific Parser
monad. The Parser
monad will encapsulate a parsing function, and we'll provide monadic operations for it through a companion object:
trait Monad[F[_]]:
def unit[A](a: A): F[A]
def bind[A, B](fa: F[A])(f: A => F[B]): F[B]
// Define the Parser monad
case class ParserResult[A](value: A, rest: String)
case class Parser[A](run: String => Option[ParserResult[A]])
object ParserMonad extends Monad[Parser]:
def unit[A](a: A): Parser[A] = Parser(s => Some(ParserResult(a, s)))
def bind[A, B](parser: Parser[A])(f: A => Parser[B]): Parser[B] = Parser { s =>
parser.run(s) match
case Some(ParserResult(value, rest)) => f(value).run(rest)
case None => None
}
In the above example:
- The
Monad
trait defines the essential monadic operationsunit
andbind
. - The
ParserResult
case class encapsulates the result of a parsing operation. - The
Parser
case class represents a parser that operates on a string and returns an optionalParserResult
. - The
ParserMonad
object provides monadic operations forParser
instances.
Basic Parsers
Next, let's define some basic parsers that will operate on characters and integers:
// Define some basic parsers
def char(c: Char): Parser[Char] = Parser { s =>
if s.nonEmpty && s.head == c then Some(ParserResult(c, s.tail))
else None
}
def int: Parser[Int] = Parser { s =>
val digits = s.takeWhile(_.isDigit)
if digits.nonEmpty then Some(ParserResult(digits.toInt, s.drop(digits.length)))
else None
}
Here, we defined two basic parsers: char
, which matches a specific character, and int
, which parses an integer from a string.
Chaining Parsers
Now, let's demonstrate how to chain these parsers together using the monadic bind
operation:
@main def ParserExample() =
val parser = given_Monad.bind(char('a')) { _ =>
given_Monad.bind(int) { number =>
given_Monad.unit(number)
}
}
println(parser.run("a123")) // Output: Some(ParserResult(123, ""))
println(parser.run("b123")) // Output: None
In the main
method, we combine a char
parser and an int
parser using the bind
method of ParserMonad
. We then run our combined parser on some input strings. This setup demonstrates how monads enable modularity and straightforward reasoning in scenarios like parsing, where operations need to be chained together in a particular sequence.
Through this example, we observe how monads facilitate the composition of operations, making our code more structured and understandable.
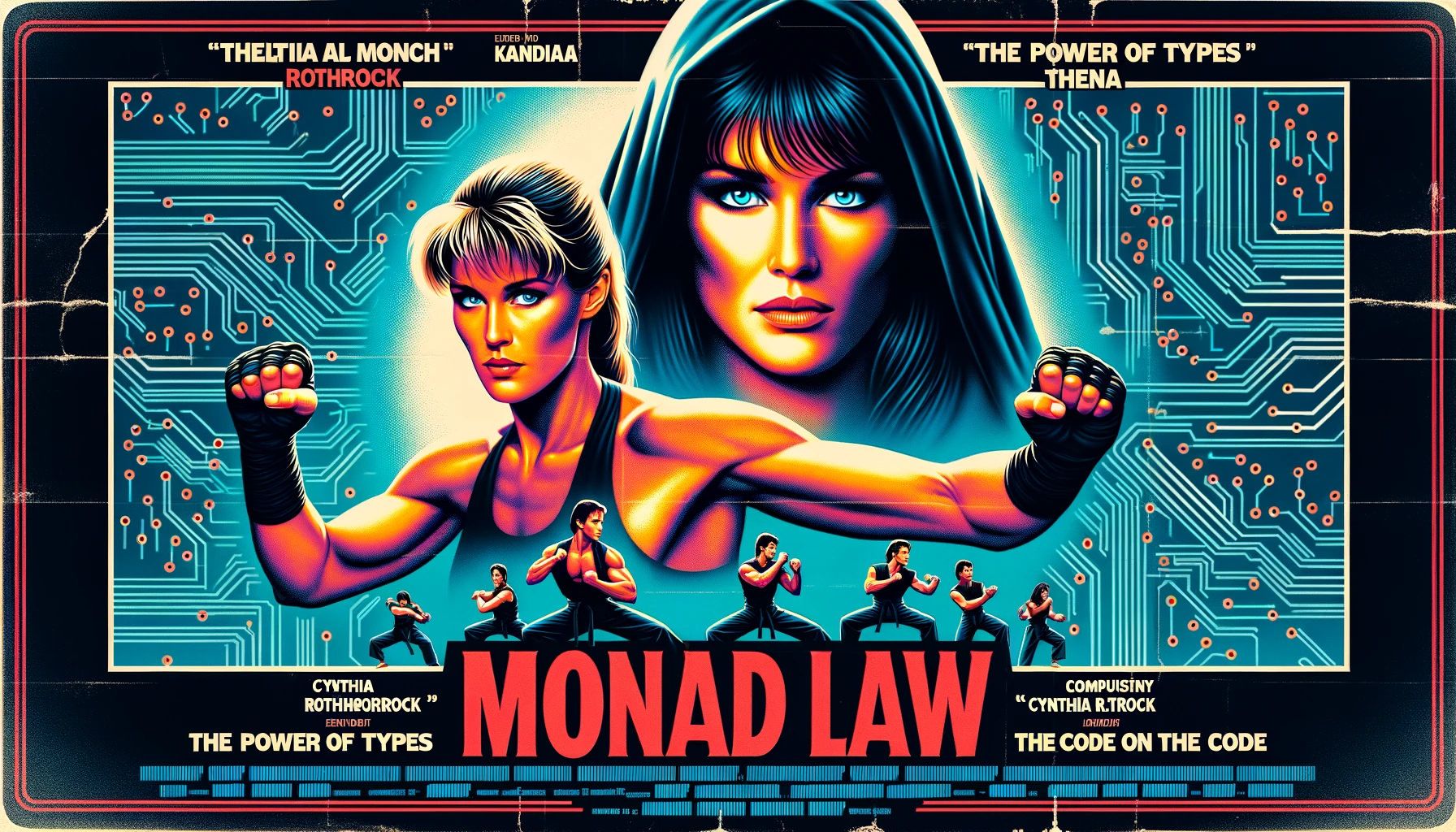
Conclusion
In this article, we've explored Monads, delving into their definition, laws, and practical implementation. Through a simple parser example, we've first learned the power they bring to managing program-wide concerns, helping us keep our code clean and understandable.
Monads, improve on Functors and Applicatives, unlocking a new level of composability and expressiveness in our code. They encapsulate a pattern that recurs in programming, making handling effects like optionality, state, or IO more structured and less error-prone.
The journey from understanding basic Functors to grasping the essence of Monads is a rewarding effort. It equips us with powerful abstractions to tackle real-world problems more structured and type-safely.
In our upcoming journey through the "Discovering Scala 3" series, we'll review these concepts again. Still, we'll use features like extension methods to write implementations that fit more naturally in our applications.
Our exploration into Monads marks not an end but a significant landmark in our ongoing adventure into the heart of functional programming and Scala.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
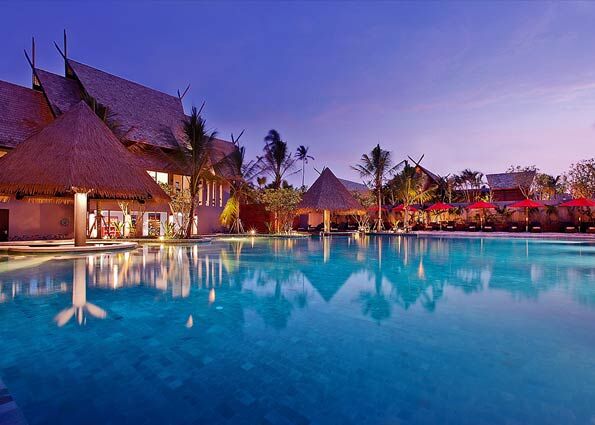
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: