Patterns of Thought: Case Analysis in Programming Languages
Explore the fundamentals of pattern matching across languages like Coq, Haskell, Scala, and Rust. Understand how case analysis improves code clarity and robustness in our multi-language exploration.
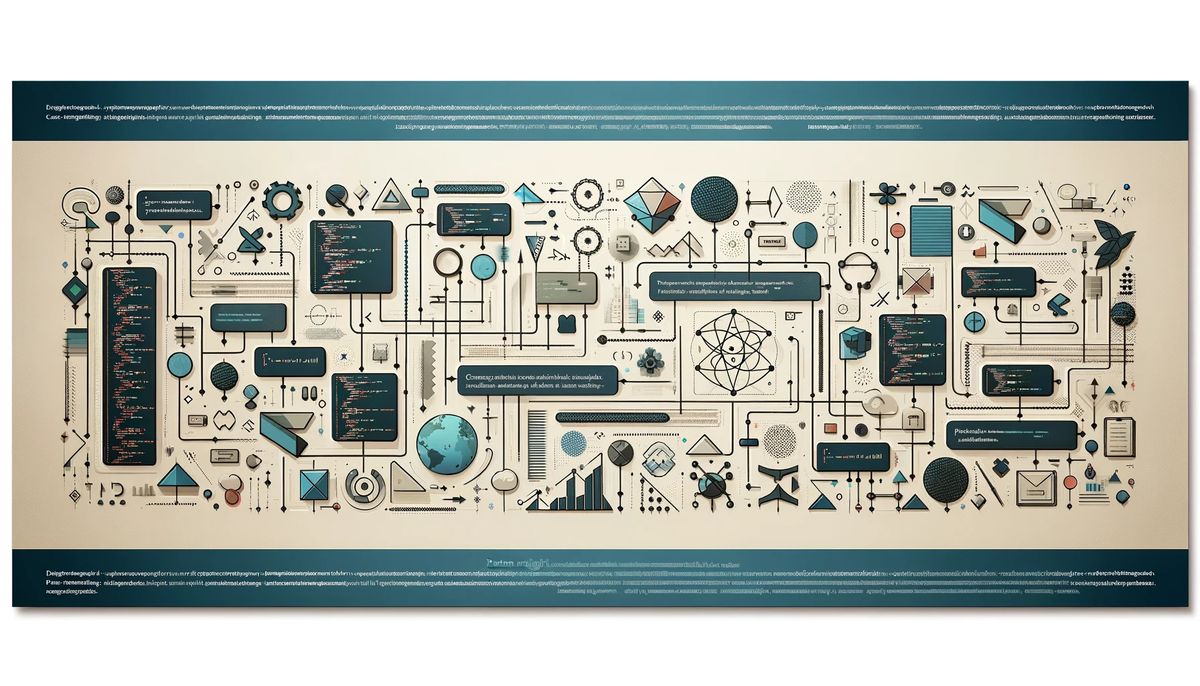
Overview: from Mathematics to Code
Case analysis has deep mathematical roots, offering a method to consider every potential outcome in a logical proof. This approach ensures the comprehensiveness of proofs and problem-solving strategies.
Theorem provers such as Agda and Coq embody the power of case analysis in verifying software correctness. They check every case to prove that an algorithm does what it is supposed to do. This thoroughness is what makes them invaluable in formal verification.
Transcending its academic origins, case analysis has become a staple in programming, particularly favored for its clarity over nested if-else chains. In programming languages like Haskell, Rust, and Scala, case analysis is a style choice and a compiler-enforced practice. Compilers in these languages can issue warnings or errors when a case is missed, guiding developers toward more exhaustive and error-resistant code.
This enforcement of case completeness aids in crafting robust and reliable software. It turns potential runtime errors into compile-time alerts, reducing bugs and enhancing code quality.
This article examines how different programming languages implement and enforce case analysis, showcasing the synergy between human foresight and compiler intelligence in creating better software.
Formal Foundations: Case Analysis in With Coq
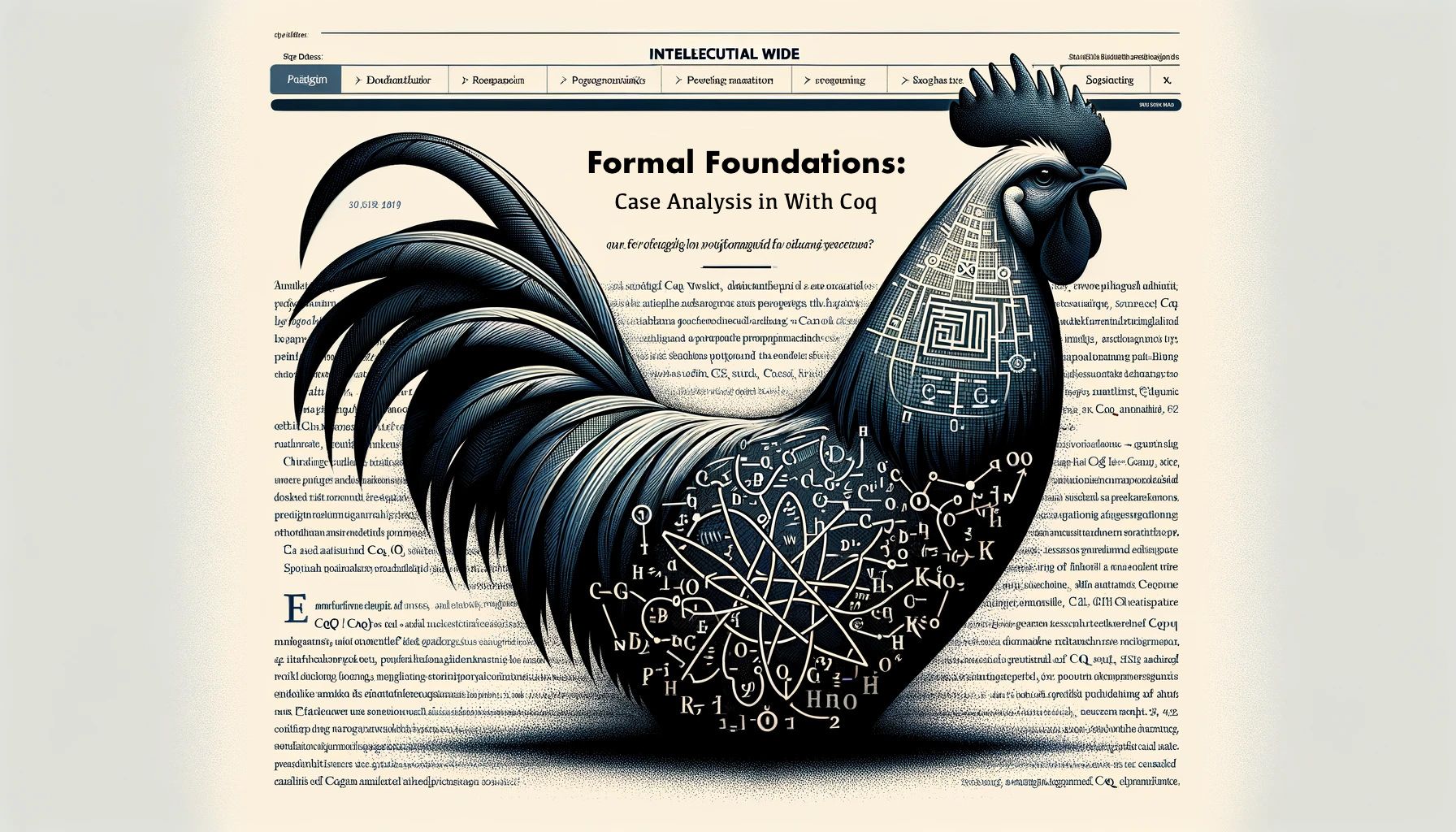
Coq stands out as a language that embodies the principle of case analysis not just as a tool, but as a foundational concept. Case analysis in Coq often starts at the very beginning of data structure definitions, leveraging an inductive approach that naturally encompasses various possibilities.
Let's see case analysis in action in the list data structure, from its definition, to formally proving a property in the reversal operation.
We start by defining the list data structure and a reverse function for that list:
Inductive list (A : Type) : Type :=
| [] : list A
| :: : A -> list A -> list A.
(* Simple list reversal without an accumulator *)
Fixpoint reverse {A : Type} (l : list A) : list A :=
match l with
| [] => []
| x :: xs => reverse xs ++ [x]
end.
The list type is defined by enumerating cases, in line 3 we defined the base case which is an empty list ([]
).
Line 4 defined the inductive case usually called cons (::
); is a constructor and slightly more complex, it's a function that takes an element and a list, then returns a new listwith the element is prepended to the list passed as a parameter.
The reversal function (reverse
in line 7) is a direct application of case analysis, it uses the match keyword and list cases for each of the possible inputs and actions, in the first case and empty list reversed is an empty list, so it returns an empty list.
The most complex case is when the list isn't empty, in that case by the definition of list will be a ::
with and element at the top and a list. In this case it reverses the smaller list without the head element, and appends that element to the end.
While the definition above is not the most efficient due to the repeated use of concatenation, which is an O(n^2) operation, it clearly demonstrates the principle of case analysis. Each case of the input is handled distinctly, and together, they cover all possible forms of the list.
Prooving That a Double Reversal Equals the Original List
A well behaved list type and reverse operation should obbey some laws, for example reversing the a list twice should result in exactly the same list.
We aim to prove the following theorem:
Theorem rev_involutive : forall (A : Type) (l : list A), reverse A (reverse A l) = l.
The theorem uses a quantifier to assert that the reversal of a reversed list should be equal to the original list, for all possible lists.
The proof involves induction on the list and case analysis on the inductive steps. Through this process, we systematically break down the problem, considering each case of the list, empty and non-empty, and applying our knowledge of the reverse
function and list concatenation.
Require Import List.
Import ListNotations.
(* Simple list reversal without an accumulator *)
Fixpoint reverse {A : Type} (l : list A) : list A :=
match l with
| [] => []
| x :: xs => reverse xs ++ [x]
end.
(* Lemma: reversing a list and appending an element is the same as appending
the element first and then reversing *)
Lemma reverse_append : forall (A : Type) (l : list A) (a : A),
reverse (l ++ [a]) = a :: reverse l.
Proof.
intros A l a.
induction l as [|x l' IHl'].
- simpl. reflexivity.
- simpl. rewrite IHl'. simpl. reflexivity.
Qed.
(* The theorem we want to prove: reversing a list twice yields the original list *)
Theorem reverse_reverse : forall (A : Type) (l : list A), reverse (reverse l) = l.
Proof.
intros A l.
induction l as [|x l' IHl'].
- simpl. reflexivity.
- simpl. rewrite reverse_append. rewrite IHl'. reflexivity.
Qed.
This proof involves induction on the list and case analysis on the inductive steps, in the lemma the cases are in 18th, and 19th. In the actual Theorem the cases are in lines 27th and 28th.
Through this process, we systematically break down the problem, considering each case, for example in line 18th we are prooving the base case for the reverse_append
lemma, which is reverse ([] ++ [a]) = a :: reverse []
, by simplifying the expression on both sides. After the simplification we get `[a] = [a]` and we invoke reflexivity completing that side of the proof.
The rest of proof is beyond the scope of this article, please feel free to leave a comment if you would like us to go into the specifics on a future article.
The proof illustrates how mathematical case analysis translates smoothly into computer science, when implemented brings a level formality and rigour hard to reproduce by other means, it ensures that each possibility is handled by our code.
Pattern Matching: Simplifying Complexity in Haskell and Scala
Pattern matching is the embodiment of case analysis in programming languages, allowing us to deconstruct data types and branch logic according to their structure. This powerful feature not only simplifies complex conditional logic but also replaces and flattens nested conditional statements, leading to more readable and maintainable code.
def processList(list: List[Int]): String = list match {
case Nil => "List is empty."
case x :: Nil => s"List has one element: $x"
case x :: xs if xs.sum > 10 => "Sum of the tail is greater than 10."
case _ => "Sum of the tail is 10 or less."
}
Haskell, a language steeped in functional tradition, integrates pattern matching into its core syntax. Through case expressions (line 3), Haskell affords programmers a powerful tool to decompose and inspect data structures.
But we can also pattern match directly in function definitions (line 12-14), providing a clear and concise way to express complex branching. It allows for immediate case analysis of input data, reducing the need for intricate if-else chains.
In contrast, Scala achieves pattern matching using the match expression (line 2). While Scala does not embed pattern matching in function signatures as elegantly as Haskell, it still effectively flattens complex logic flows into readable and exhaustive case analyses.
Pattern matching thus represents a fundamental tool for case analysis in both Haskell and Scala, enabling succinct, expressive, and reliable branching in code without the pitfalls of deep conditional nesting. It's an approach that leverages the compiler's power to enforce exhaustiveness, further strengthening the reliability of the code.
We've just scratched the surface of what pattern matching can do. In future articles, we will delve deeper into the advanced pattern matching features of Scala and Haskell. From the elegance of Scala's extractors that allow for infinitely customizable patterns to Haskell's views, there's a rich tapestry of features to explore.
Pattern Matching in Rust: Precision and Safety
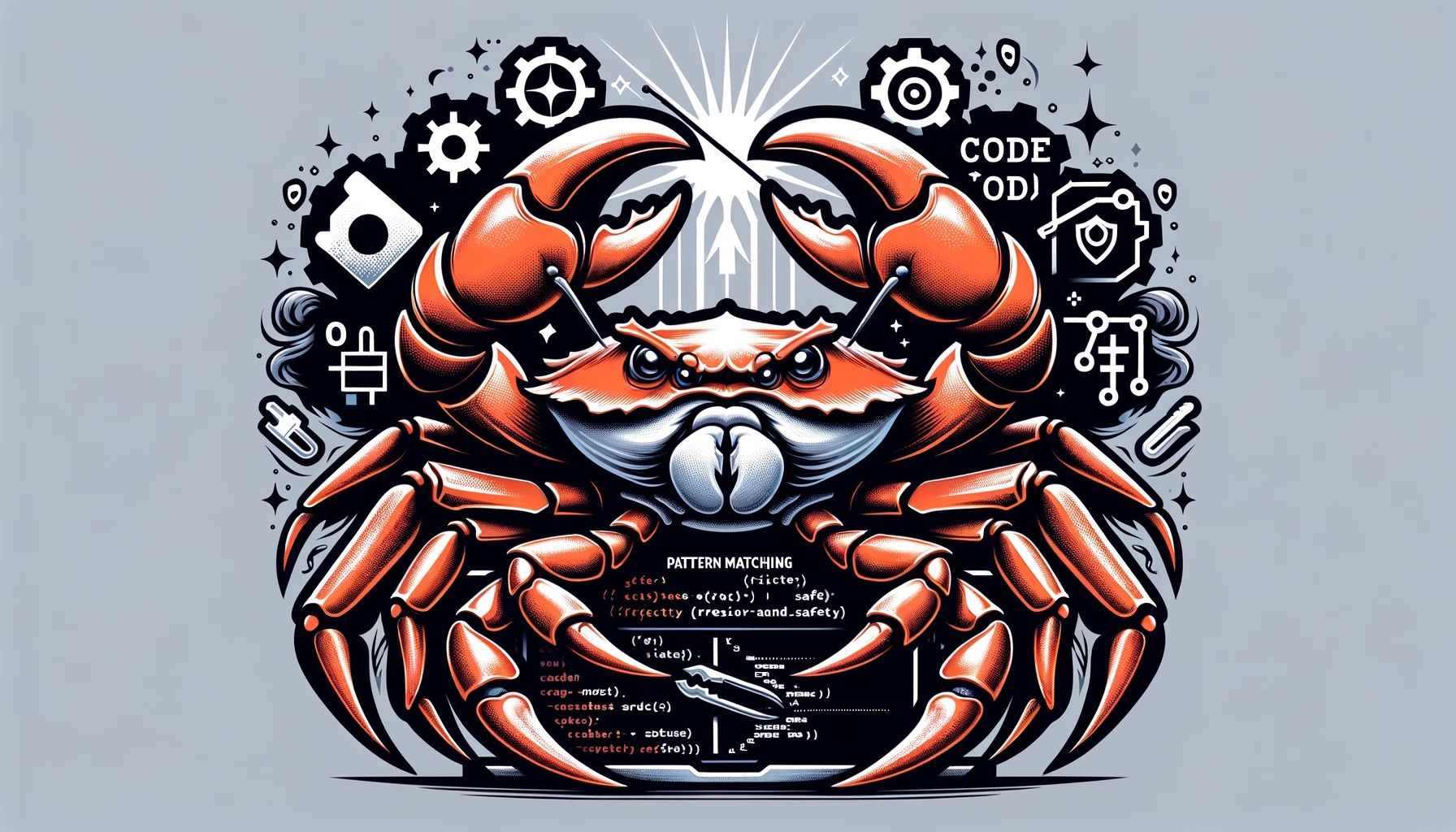
Rust, a language known for its focus on safety and performance, incorporates pattern matching as a cornerstone of its syntax, much like its functional predecessors.
In Rust, pattern matching is not just a convenience, it's a requirement for certain operations, ensuring that all possible cases are handled, thus preventing bugs and crashes that might arise from unhandled cases.
Here's a glimpse at Rust's pattern matching in action:
fn main() {
let value = Some(7);
match value {
Some(x) if x < 5 => println!("Found a small number!"),
Some(x) => println!("Found a number: {}", x),
None => println!("Found nothing!"),
}
}
Rust's compiler enforces exhaustive checking, making sure that every possible case is covered when using match
. This, combined with Rust's strict type system and ownership model, makes pattern matching a robust tool for developers.
With Rust, pattern matching extends beyond simple value matches. It includes powerful features such as:
- Guards for adding additional conditions to patterns.
- Bindings, to not just check the pattern, but also to bind values to names for further use.
- Destructuring, to break apart complex data types and match on their contents directly.
The exhaustive nature of pattern matching in Rust aids in writing reliable code that's resilient to edge cases, offering guarantees at compile time that other languages might only catch at runtime—or worse, not at all.
Rust demonstrates how pattern matching can be a fundamental construct in a systems programming language, contributing to the language's goal of zero-cost abstractions without sacrificing performance or safety.
Python Joins the Fray
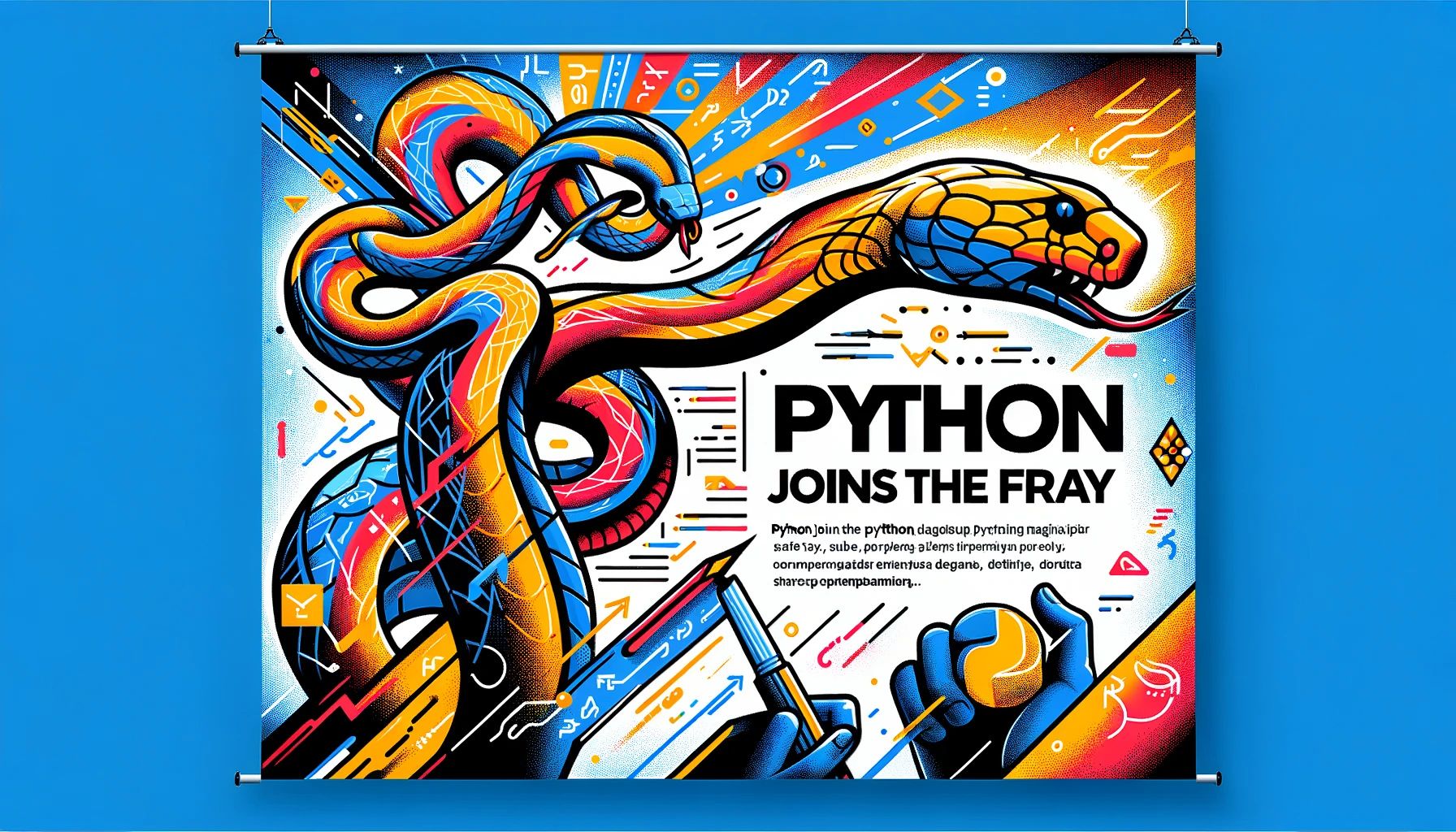
While pattern matching has been a long-standing feature in functional languages, other programming paradigms have also begun to adopt this powerful construct. Python, a language revered for its simplicity and readability, introduced structural pattern matching in version 3.10, embracing the functional paradigm's influence.
With the addition of the match
statement, Python programmers can now enjoy a more expressive syntax for conditional logic. Here's how Python's pattern matching can simplify code:
def process_list(ls):
match ls:
case []:
return "List is empty."
case [x]:
return f"List has one element: {x}"
case [x, *xs]:
sum_tail = sum(xs)
if sum_tail > 10:
return "Sum of the tail is greater than 10."
else:
return "Sum of the tail is 10 or less."
Python's adoption of pattern matching is a testament to the utility and versatility of this feature across different programming paradigms. It's a move that encourages developers to think more functionally, showing how concepts from functional programming can inform and improve practices in a broader programming context.
Conclusion
Pattern matching is a powerful feature that simplifies code by replacing complex conditional logic. It's present in languages like Coq, Haskell, Scala, and Rust, each adding flavor to this concept. This feature ensures the code is concise and more maintainable while allowing compilers to check for missing logic paths.
As we wrap up, it's obvious pattern-matching is a significant step towards more robust and less error-prone software development across various programming paradigms. Our journey through different languages highlights the adaptability and crucial role of pattern matching in modern coding practices.
Keep an eye out for more deep dives into specific pattern-matching features in future articles, where we'll explore the intricacies and advanced uses in different programming languages.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
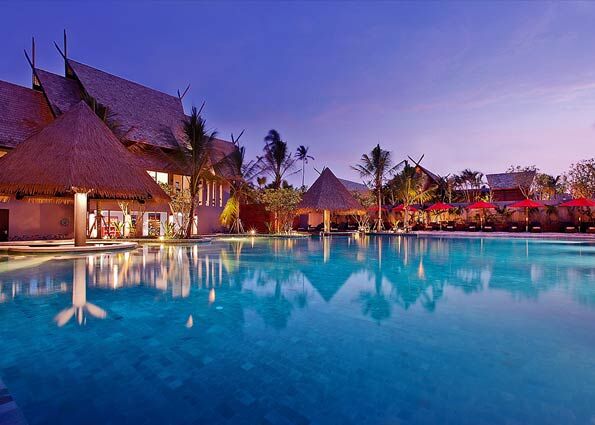
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: