Python Unpacked: From Tuple Assignment to Pattern Matching (2)
Explore Python's unpacking with PEP 448 and Typed Dictionaries. Master advanced Python features for better code clarity and type safety. Subscribe for more Python tips and updates. Please make sure to enhance your Python skills.
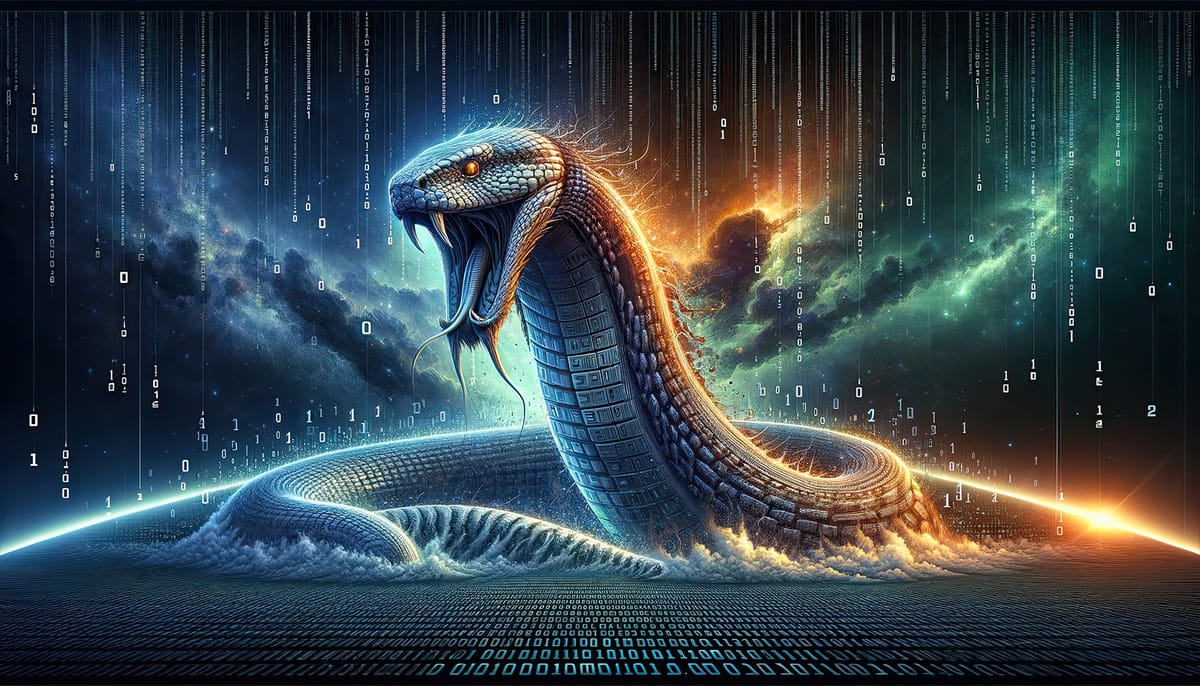
Overview
In our previous exploration, we embarked on a historical journey through Python's data handling evolution, from the rudimentary yet foundational tuple assignment to the sophistication of iterable unpacking.
Today, we delve further into this narrative, starting with dictionaries and keyword arguments, moving to the extended unpacking capabilities introduced in Python 3.5 with PEP 448, and ending with introducing type safety to keyword arguments using Typed Dictionaries.
These advancements have refined Python's versatility in data handling and opened new avenues for writing more precise, more efficient code. Join us as we unravel these developments, showcasing their practical applications and profound impact on Python programming.
Dictionaries, kwargs, and Basic Unpacking in Python
Dictionaries in Python have long been a staple for managing data efficiently. These structures, characterized by key-value pairs, offer an intuitive means to store and retrieve data by name. For instance, creating a dictionary to hold a person's information is straightforward:
person_info = {'name': 'John', 'age': 30}
print(person_info['name']) # Outputs: John
In this example, person_info
is a dictionary with keys 'name' and 'age', allowing easy access to each piece of data.
The concept of **kwargs
(keyword arguments) is closely tied to dictionaries. This feature in function definitions enables us to pass a variable number of named arguments. These arguments are then accessible as a dictionary within the function, allowing for flexible and dynamic handling of inputs. For example:
def print_info(**kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
print_info(name='Alice', age=25, city='New York')
print_info
can accept any number of named arguments, printing out each key-value pair. This flexibility is a hallmark of Python's design, emphasizing readability and efficiency.
Before introducing PEP 448 in Python 3.5, dictionary unpacking was already a feature, albeit in a more limited scope. It was primarily used to pass dictionaries as keyword arguments in function calls. The **
operator would unpack the dictionary, mapping its keys and values to the corresponding parameters of the function:
def register_user(name, age, country):
print(f"Name: {name}, Age: {age}, Country: {country}")
user_data = {'name': 'Emma', 'age': 35, 'country': 'Canada'}
register_user(**user_data)
In this example, user_data
is unpacked by **
, and its contents are passed as arguments to register_user
. This approach provided a way to handle dynamic arguments but was limited to function calls and did not allow for direct merging or manipulation of dictionaries.
In Python, when using dictionary unpacking with **
in function calls, the names of the parameters in the function definition are used to match the correct values from the dictionary. The order of elements in the dictionary does not matter; the keys matter. The keys in the dictionary must match the names of the parameters in the function's signature.
For example, in the register_user(**user_data)
function, user_data
is a dictionary with keys name
, age
, and country
. These keys correspond to the parameter names in the register_user
function. Python matches the dictionary keys with the function parameters by name, not by order, ensuring that the correct values are assigned to the respective parameters.
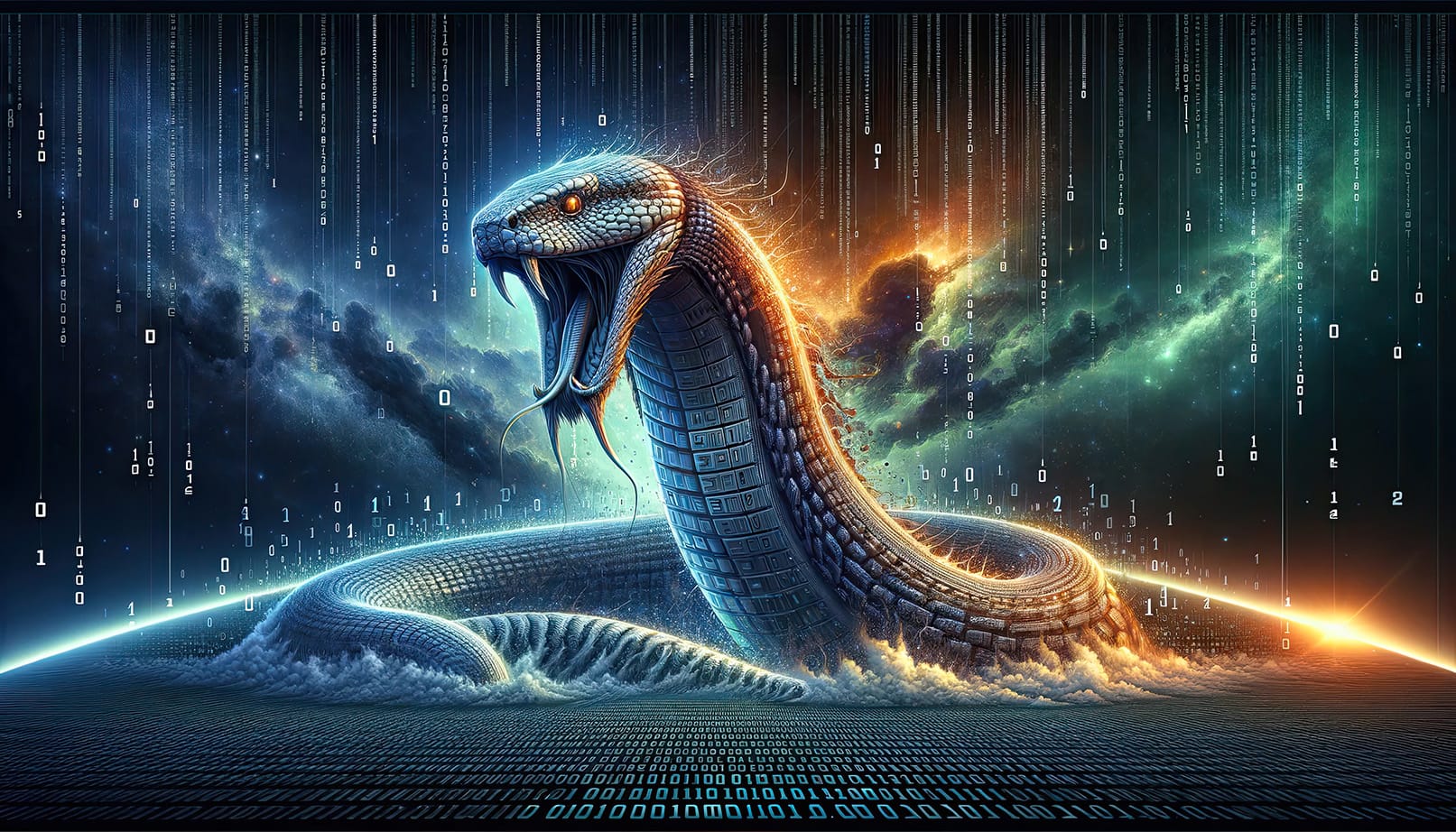
PEP 448: Broadening the Horizons of Unpacking in Python
Introduced in Python 3.5, PEP 448 significantly broadened the unpacking capabilities of Python, enhancing not only how dictionaries are handled but also enriching the unpacking of other iterables. This PEP stands out for introducing several key features.
One notable enhancement is the extended unpacking in literals, allowing elements of iterables like lists and sets to be unpacked more expressively. For instance, you can combine lists with ease. Merging dictionaries became more streamlined as well. With the use of the **
operator, merging multiple dictionaries into a single one is now a concise operation:
list1 = [1, 2]
list2 = [3, 4]
combined = [*list1, *list2] # Results in [1, 2, 3, 4]
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged = {**dict1, **dict2} # Merges dict1 and dict2
The addition of stable ordering to dictionaries, with PEP 468 in Python 3.7, made unpacking dictionaries to variables practical.
When unpacking a dictionary into variables, Python assigns keys (or values if specified) in the order they appear in the dictionary, not based on the variable names:
my_dict = {'a': 1, 'b': 2, 'c': 3}
key1, key2, key3 = my_dict # Unpacks keys
# Unpacks values
value1, value2, value3 = my_dict.values()
# Converts key-value pairs into a list of tuples
key_value_pairs = list(my_dict.items())
merged_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
(a, v1), (b, v2), (c, v3), (d, v4) = merged_dict.items()
# a, b, c, d are the keys
# v1, v2, v3, v4 are the corresponding values
As line 11th demonstrates, Python allows us to write sophisticated unpacking of dictionary items into variables. This can be done by unpacking the .items()
method of the dictionary, which returns key-value pairs as tuples. We can use an underscore _ instead of a variable name at any position to ignore the value, as we can do when unpacking any other data structure.
Using Typed Dictionaries in Python
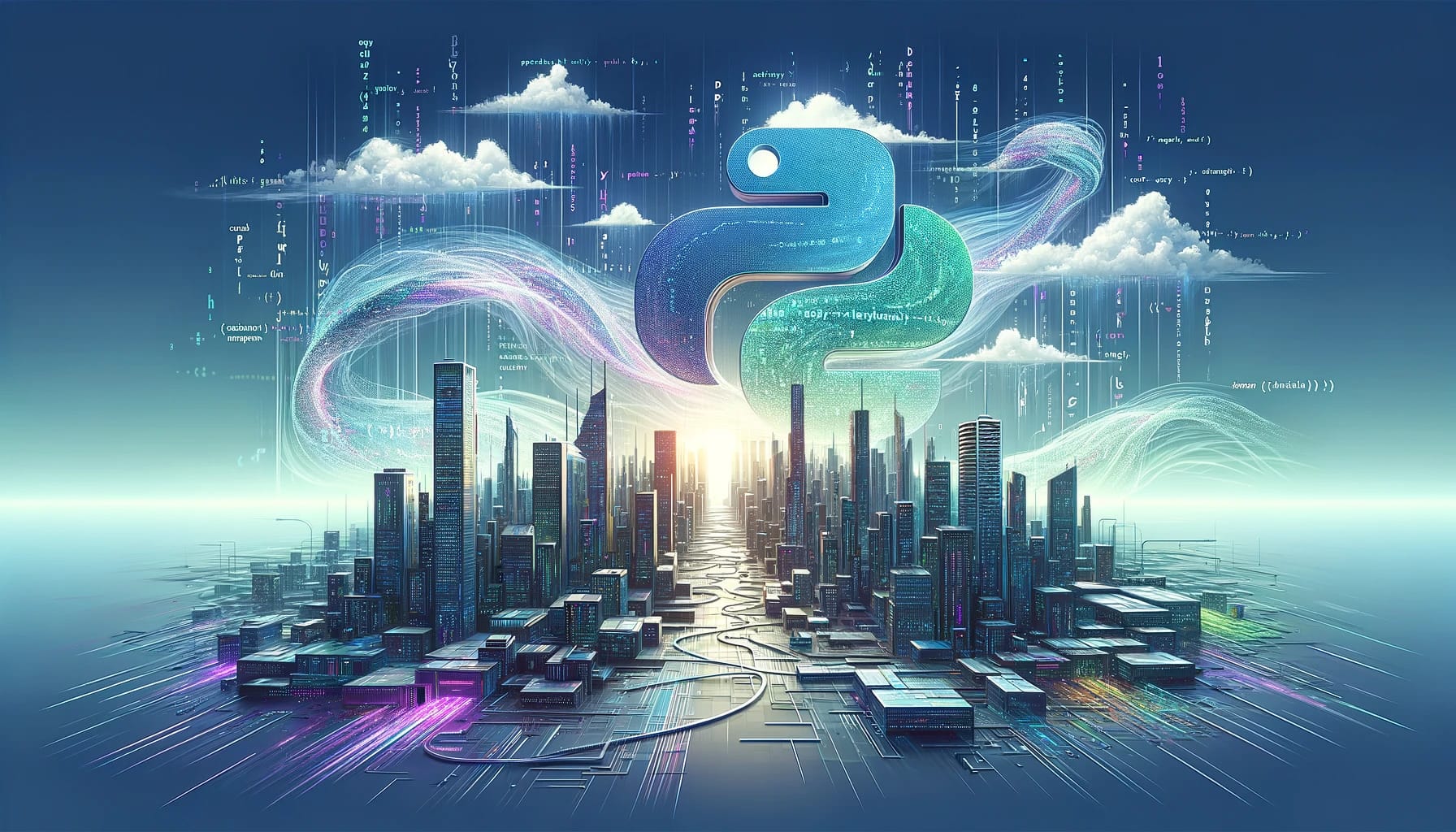
In modern Python development, typing enhances code clarity, maintainability, and error prevention. As explored in Turing Taco's article on Type Hints, type annotations in Python are a powerful tool against code anarchy, providing developers with a clearer understanding of the codebase and reducing the likelihood of runtime errors.
The introduction of Typed Dictionaries, formalized in PEP 589, enables us to define expected types for keys and values within a dictionary. This feature is especially beneficial in codebases where dictionaries are central to data handling and structure.
Typed Dictionaries were further refined by PEP 468 in Python 3.12. With this improvement, they can now be used with the **
operator, allowing us to ask Python to unpack them as named arguments in function calls while maintaining type safety with a tool like mypy
:
from typing import TypedDict
# Defining the Typed Dictionary
class Person(TypedDict):
name: str
age: int
# Function that takes named arguments
def greet(name: str, age: int):
print(f"Hello, {name}! You are {age} years old.")
# Creating an instance of the typed dictionary
alice: Person = {'name': 'Alice', 'age': 30}
# Using ** to unpack the dictionary into named arguments
greet(**alice)
This code is type-safe:
Person
is a Typed Dictionary with specified types for the 'name' and 'age' keys.- The
greet
function is defined to take two named arguments,name
andage
. - An instance of
Person
,alice
, is created, conforming to the Typed Dictionary's structure. - The
**
operator is used to unpackalice
into named arguments when callinggreet
. This ensures that the keys in thePerson
dictionary match the parameter names of thegreet
function.
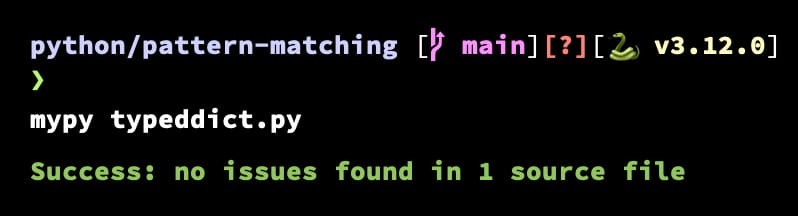
mypy
The benefits of using Typed Dictionaries are manifold. They bring the advantages of static typing to a traditionally dynamic data structure, enhancing type safety and reducing the risk of type-related bugs. Additionally, they improve the readability and maintainability of the code, particularly in complex projects where understanding data structures is crucial.
Incorporating Typed Dictionaries into a Python project aligns with the broader trend towards more structured and type-safe code, a shift gaining momentum in the Python community.
Conclusion
In conclusion, the advancements in Python's unpacking capabilities, particularly with PEP 448, and the introduction of Typed Dictionaries have significantly enhanced the language's expressiveness and type safety. These features exemplify Python's evolving nature, continually adapting to provide more robust and precise coding practices.
Typed Dictionaries, especially when combined with the unpacking syntax, offer a powerful tool for developers. It ensures the clarity of data structures and enforces type consistency across function interfaces. This is a significant step towards making Python codebases more maintainable, readable, and less prone to errors.
As Python continues evolving, we should leverage these capabilities in large-scale applications and small scripts to write cleaner, more efficient, and reliable code.
If you've found these insights into Python useful, take advantage of future updates and explorations into the world of Python and its evolving features. Subscribe to our publication for more articles like this. Continue your journey into programming with us! 🐍🚀
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
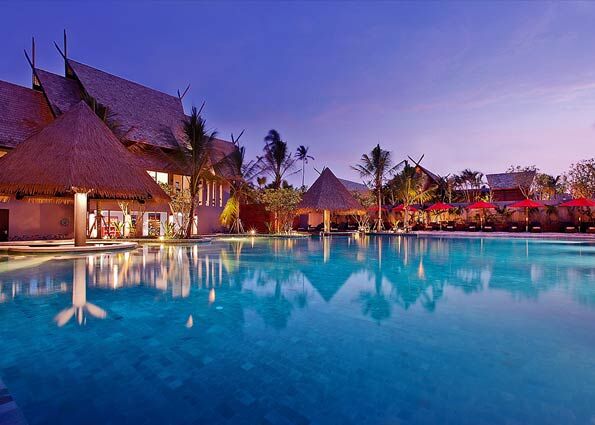
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: