Python Unpacked: From Tuple Assignment to Pattern Matching (1)
Explore Python's evolution in unpacking techniques, from tuple assignments to extended unpacking. Learn how Python's versatility shapes coding practices. Stay tuned for Part 2! Subscribe for updates.
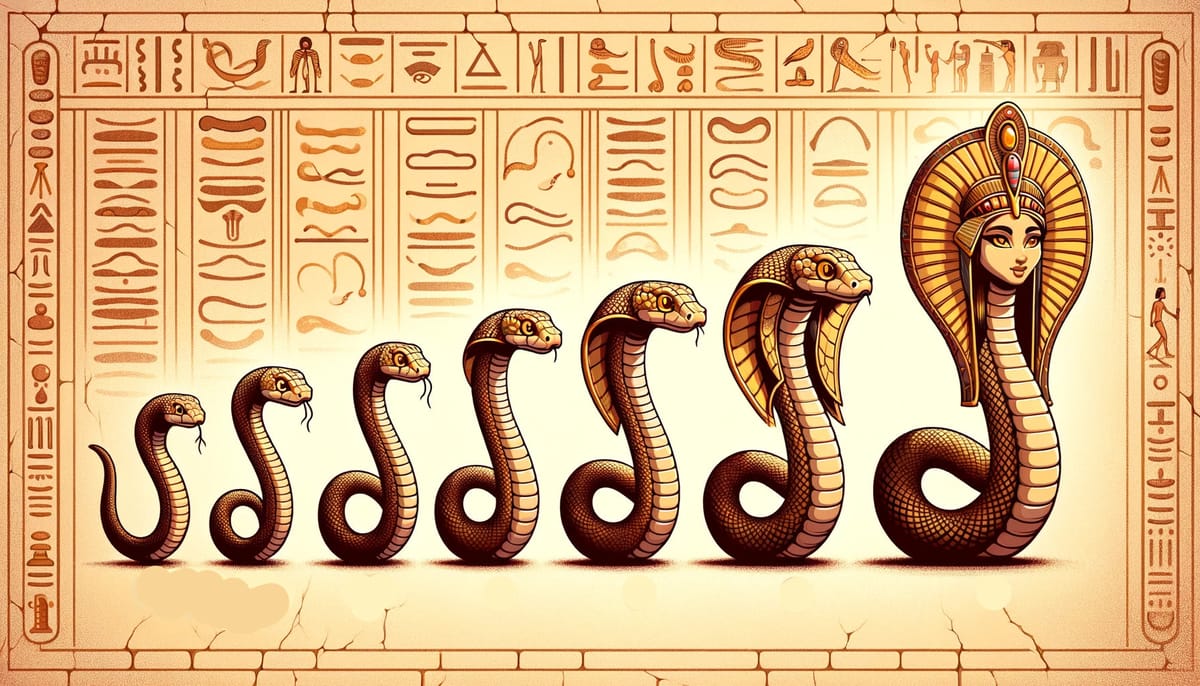
Overview
In this article, we embark on a historical adventure, tracing Python's evolution in data handling. A journey through Python's history that reveals how each feature addition has been a response to the evolving needs of its programming community.
Our starting point is the humble yet foundational tuple assignment; think of it as the language's Stone Age: essential, functional, and crucial to handling data.
Then, we move forward through the Bronze Age, with the advent of unpacking. Unpacking brought new sophistication and flexibility, allowing programmers to handle data more easily and quickly.
The Iron Age brought further refinements to the unpacking mechanism (PEP 448), removing restrictions and allowing unpacking to happen in more places of a program.
This led to the modern era of Python with structural pattern matching, a Renaissance in data handling. We covered this renaissance in depth in our previous articles: "Python’s Power Play: Structural Pattern Matching", "Putting the Structural in Structural Pattern Matching", and finally, "Pattern Matching: Lists and Dictionaries in Python".
Understanding this historical progression offers crucial insights into Python's design philosophy. It helps us appreciate why certain features exist, how to use them effectively and imagine future developments.
Join us in this exploration to understand Python's evolution and how it shapes our code today.
Python's Data Handling Origins: Tuple Assignment
Our journey through Python's data handling evolution begins with a foundational feature: tuple assignment. Present since Python 1.0, this feature set the tone for the language's enduring commitment to simplicity and readability.
Early Python's Simple Yet Powerful Tool
Since its introduction in Python 1.0, tuple assignment has been a staple in Python programming:
x, y = 10, 20
This straightforward yet effective method allowed for assigning multiple values to multiple variables simultaneously, showcasing Python's early inclination towards clear and concise code.
Tuple assignment represents the starting point of Python's journey in intuitive data handling. It demonstrated early on how Python prioritizes reducing complexity and enhancing code clarity.
As the earliest tools were essential for developing more advanced technologies, tuple assignment was crucial for Python's progression towards more sophisticated data handling capabilities. This feature laid the groundwork for the language's future innovations, paving the way for developing more complex and versatile features.
From Tuples to Iterables
Python's initial unpacking feature was limited to tuples, allowing the initialization of multiple variables by assigning them to a tuple. As Python evolved, developers requested the extension of this feature to support iterable objects in general:
# Unpacking a tuple
n, s = [1, "this is not a string"]
# Unpacking a list
a, b, c = [1, 2, 3]
# Unpacking a string
x, y, z = "XYZ"
# Unequal length iterable and variables
# (Throws "ValueError: not enough values to unpack (expected 3, got 2)")
a, b, c = [1, 2]
# Inability to capture surplus elements
# (Throws "ValueError: too many values to unpack (expected 2)"")
x, y = [1, 2, 3]
Despite its versatility, iterable unpacking had similar constraints to tuple unpacking; the number of variables on the left side had to match the number of data elements on the right side of the equal sign. In the above example, lines 9th and 13th show attempts to unpack an iterable with an unequal number of elements or not capturing surplus aspects, which will result in ValueError
. This rigidity made the feature unusable for dynamically sized data structures.
The Next Evolution: Extended Unpacking
The introduction of extended unpacking in Python marked a significant milestone in the language's evolution, offering enhanced capabilities for handling iterable objects. This feature was introduced with PEP 3132 and became available in Python starting from version 3.0. Let's explore what PEP 3132 added and how it expanded Python's unpacking capabilities.
PEP 3132 extended unpacking to Python, enabling more versatile unpacking of iterable objects. This enhancement allowed developers to extract and manipulate elements from sequences and iterables with greater flexibility and precision.
One of the key features introduced by PEP 3132 is the ability to use the star operator (*
) in iterable unpacking. While the star operator was already known for its role in variable-length argument lists (e.g., *args), this PEP extended its functionality to iterable unpacking, opening up a world of possibilities.
The Star Operator (*
): Unleash the Power
Python's star operator (*) is a versatile tool that takes unpacking to the next level. While it was initially introduced to handle variable-length argument lists in function definitions (known as *args), it quickly found its way into other aspects of the language, including unpacking iterable objects.
The star operator can be used in various contexts, but its primary role in unpacking is to gather or spread elements. When used in unpacking, it enables you to:
- Gather Elements: Collect multiple elements of an iterable into a single variable.
- Spread Elements: Distribute elements of a variable into multiple individual variables.
Let's dive into some examples to illustrate these concepts.
Gathering Elements with the Star Operator
Suppose you have a list of numbers, and you want to extract the first number and gather the rest into a separate list:
numbers = [1, 2, 3, 4, 5]
first, *rest = numbers
print(first) # Output: 1
print(rest) # Output: [2, 3, 4, 5]
This example first
captures the first element and *rest
gathers the remaining elements into a list.
Spreading Elements with the Star Operator
Conversely, you can use the star operator to spread elements from an iterable into individual variables. This is especially useful when dealing with functions that accept a variable number of arguments:
def multiply(a, b):
return a * b
args = (2, 3)
result = multiply(*args)
print(result) # Output: 6
Here, args
is a tuple containing two values, and we use the star operator to spread those values as arguments to the multiply
function.
Nesting and Nested Unpacking
Nesting refers to placing one or more iterable objects within another, creating a hierarchical or nested structure. Python allows you to nest various data structures, such as lists, dictionaries, or combinations. Nested structures are often encountered when dealing with complex data, such as JSON objects or multi-level lists.
The star operator becomes especially useful when working with nested structures, as it can simplify extracting values from deeply nested objects. Here's an example of nested unpacking:
nested_list = [1, [2, 3], [4, [5, 6]]]
first, (second, third), (fourth, (fifth, sixth)) = nested_list
print(first) # Output: 1
print(second) # Output: 2
print(third) # Output: 3
print(fourth) # Output: 4
print(fifth) # Output: 5
print(sixth) # Output: 6
In this example, we have a deeply nested list and use nested unpacking to access values at different levels within the structure.
Handling Errors with Nested Unpacking
Being cautious when working with nested unpacking is essential, as unexpected structures or missing values can lead to errors. To handle such situations gracefully, you can use try-except blocks to catch exceptions that may arise during the unpacking process:
nested_data = [1, [2, 3], [4, [5, 6]]]
try:
first, (second, third), (fourth, (fifth, sixth), seventh) = nested_data
except ValueError as e:
print(f"Error: {e}")
In this example, an error occurs because we're trying to unpack the seventh
, which doesn't exist in the nested structure. A try-except block allows us to handle such cases without crashing the program.
Conclusion
We've seen how Python's commitment to versatility and readability has led to the development of powerful unpacking techniques that enhance our coding experience. From the humble beginnings of tuple assignment to introducing the star operator and beyond, Python continues to evolve, providing us with tools to handle iterable objects with finesse.
But the story continues. In the next installment of our series, we'll closely examine the latest advancements in Python unpacking.
If you've enjoyed this journey through Python's unpacking history, please subscribe to our publication. We appreciate your support and look forward to bringing you more insights into Python's ever-evolving features and techniques. Subscribe now and join us in exploring the exciting world of Python!
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
In the meantime, I'll be accepting donations. I would appreciate it if you consider a donation, provided you can afford it:
Every donation helps me offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated.
Also, if you are looking for a fancy way to receive 2025, I am renting my timeshare in Phuket to cover part of the unexpected costs.
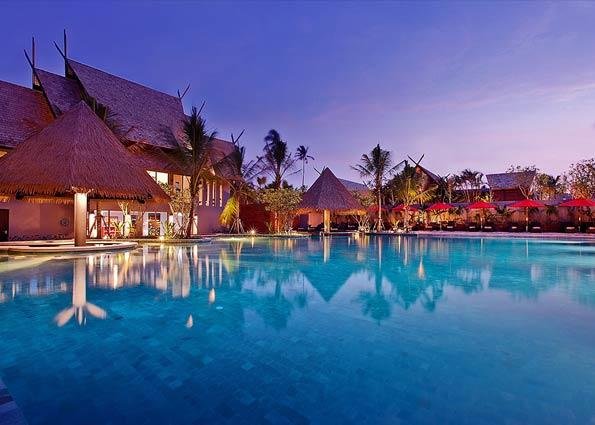
Or share our articles and links page in your social media posts.
Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble.
Take a look. Maybe you can find something you like: