Python’s Power Play: A Structural Pattern Matching First Look
Explore the transformative power of structural pattern matching in Python 3.10 with 'Pimp My Code'. Discover how this feature revolutionizes data handling, enhances code clarity, and makes implementing Algebraic Data Types more practical. Embrace modern Python programming with this guide.
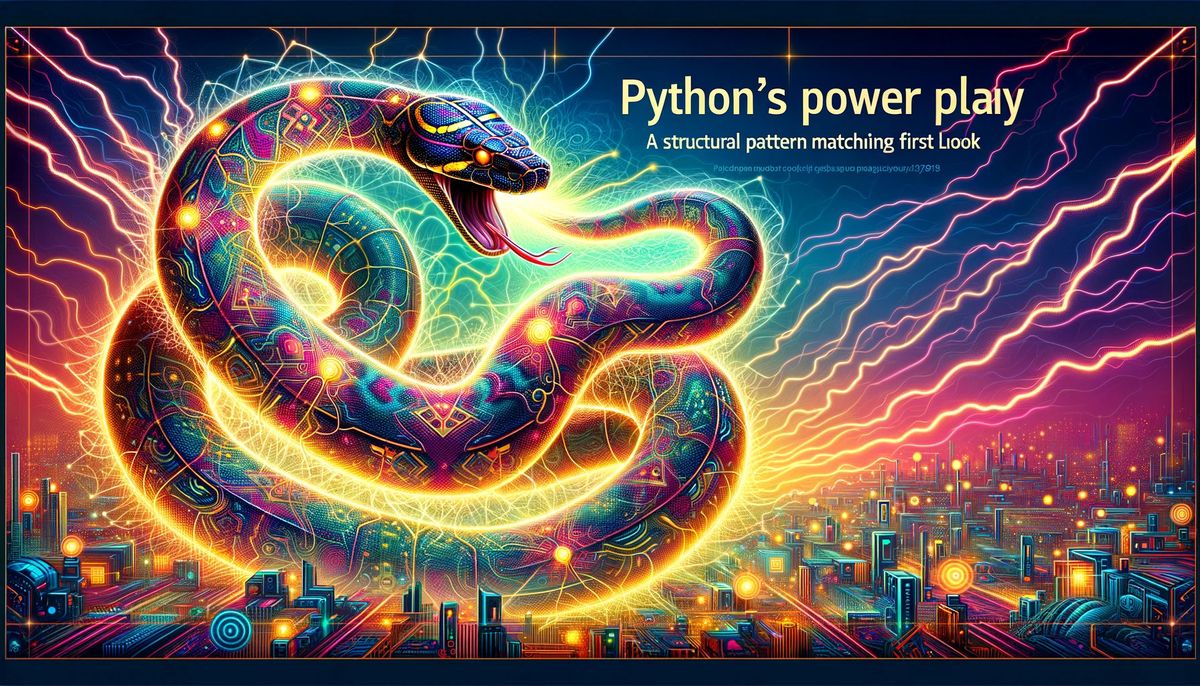
Overview
In "Patterns of Thought: Case Analysis in Programming Languages", we explored how some programming languages adopted case analysis from mathematics, gaining expressiveness, clarity, and exhaustiveness safety. In most programming languages, case analysis is called pattern matching.
Until Python 3.10, developers had to deal with several disadvantages because of this lack of adoption of pattern matching in the language:
- Complex Conditional Logic: Without pattern matching, conditional logic often meant chaining multiple
if-else
statements to process different data scenarios. And in the worst case, deep nesting of the code makes it hard to read. - Increased Risk of Errors: Manual data extraction and checks were prone to errors. Requiring additional code to ensure data integrity, more manually written code means more opportunities for mistakes and oversights.
- Lack of Intuitive Code Structure: Case analysis is easy to understand and has a clear structure, where we describe the case and then write the code to handle that case. The absence of pattern matching meant expressing complex data handling logic in more convoluted (chained conditionals) or dispersed cases (virtual dispatching).
- Verbose Data Unpacking: Extracting data from complex structures like nested lists or dictionaries requires multiple steps and lines of code.
- Limited Code Readability and Maintenance: As the complexity of data operations increased, the readability and maintainability of the code decreased. The lack of pattern matching led to more boilerplate code, making it challenging for other developers to read and modify.
These disadvantages are significantly mitigated by introducing pattern matching in Python 3.10. The feature brings new clarity, efficiency, and expressiveness to Python, making it easier for developers to work with complex data while keeping the code readable and maintainable.
Introducing the Example: The Crypto Watcher Script
In the script above, written without using pattern matching, we can observe several disadvantages that were typical before Python 3.10:
- Complex Conditional Logic (Lines 26-37): The function
process_data
relies on nestedif-else
statements to handle different data scenarios based on the volume and market cap. This nesting results in complex and less readable code, a common challenge when dealing with various data types and structures. - Increased Risk of Errors (Lines 26-37): the conditional blocks check condition on the data points (
volume
,market_cap
). Such manual checks increase the risk of errors, requiring careful validation of each condition and the data's structure. - Verbose Data Unpacking (Lines 42-44, 48-50): The data extraction from the API response (
data['prices'][-1][1]
,data['total_volumes'][-1][1]
) is multi-step and verbose. Pattern matching would allow for a more streamlined and concise unpacking of these nested structures. - Limited Code Readability and Maintenance (Entire Script): The script, while functional, demonstrates how the lack of pattern matching can lead to more boilerplate and less intuitive code, especially when processing and interpreting data from an external API. This can impact the overall maintainability and readability of the code.
The absence of pattern matching in Python led to more cumbersome and less efficient code.
Pimp My Code With Structural Pattern Matching
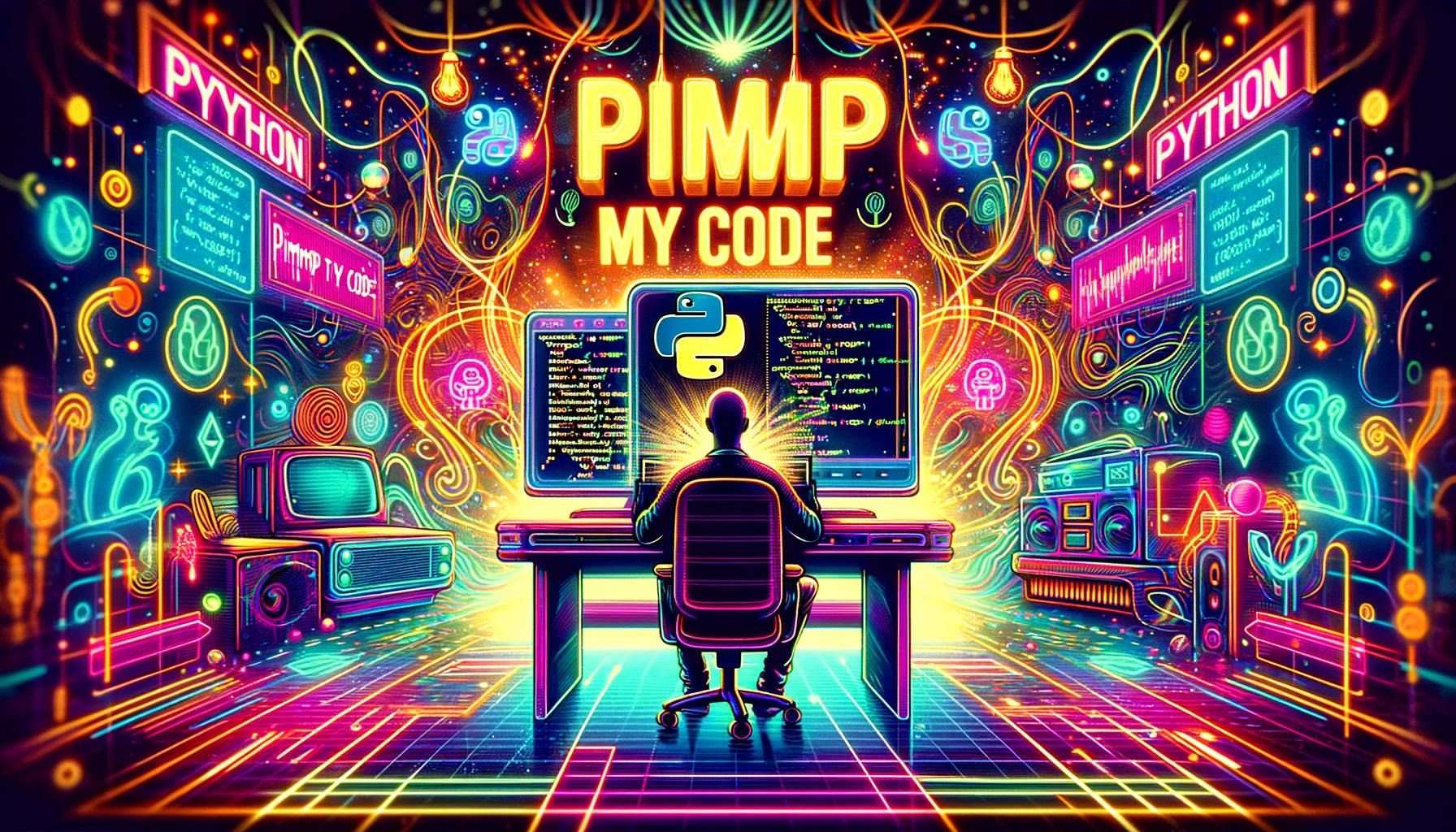
The introduction of structural pattern matching in our crypto watcher script revolutionizes how the data is processed. Let's explore the key improvements:
- Simplified Conditional Logic: With pattern matching, the previously complex nested
if-else
statements in theprocess_data
function are replaced by clear and concisematch
statements. This simplification makes the logic more straightforward and the code easier to follow. - Direct Data Mapping: The script now directly maps the data structure returned from the API to variables within the
match
case statements. This direct mapping allows for a more intuitive approach to extracting and working with specific pieces of data, likeprice
,market_cap
,volume
, andchange
. - Improved Readability and Maintainability: The pattern-matching syntax leads to more readable code. Each
case
clearly defines the criteria for the data it handles, making the script more maintainable and straightforward for other developers to understand and modify. - Efficient Data Handling: The script becomes more efficient in handling different data scenarios. Pattern matching provides a structured and elegant way to manage multiple conditions without requiring lengthy, error-prone manual checks.
- Enhanced Error Handling and Default Cases: Including a default case (
case _:
) in the pattern matching ensures that the script gracefully handles unexpected data formats or missing data, enhancing the robustness of the script.
These enhancements demonstrate the power of structural pattern matching in Python. It's not just about writing less code but more expressive, readable, and maintainable code. This approach aligns with modern programming practices, where clarity and simplicity are essential, especially when dealing with complex data structures, as is often the case in API responses.
Conclusion
Introducing structural pattern matching in Python is a familiar feature. It's a gateway to more expressive and efficient coding practices.
By simplifying the handling of complex data structures, pattern matching makes the implementation of Algebraic Data Types (ADTs) more practical and powerful in Python, as detailed in "Python’s Path to Embracing Algebraic Data Types" on TuringTaco.
This marks a significant stride in Python's evolution, aligning it with the advanced capabilities of functional programming languages. Unfortunately, Python implemented it as a statement instead of an expression, which makes its application cumbersome in some situations.
Python's Pattern Matching implementation can do much more than we cover in this first look. Embrace this change and unlock new possibilities in your Python projects.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
In the meantime, I'll be accepting donations. I would appreciate it if you consider a donation, provided you can afford it:
Every donation helps me offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated.
Also, if you are looking for a fancy way to receive 2025, I am renting my timeshare in Phuket to cover part of the unexpected costs.
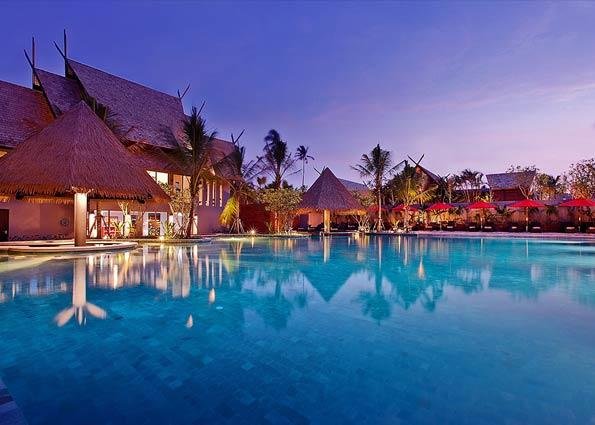
Or share our articles and links page in your social media posts.
Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble.
Take a look. Maybe you can find something you like: