The Scala Way: Understanding Expressions and Statements
Explore our latest mini-article, a supplement to our Scala 3 series. Unravel the nuances of Scala's approach to expressions and statements, a critical feature that simplifies the language and enhances code clarity. Perfect for both beginners and seasoned Scala developers.
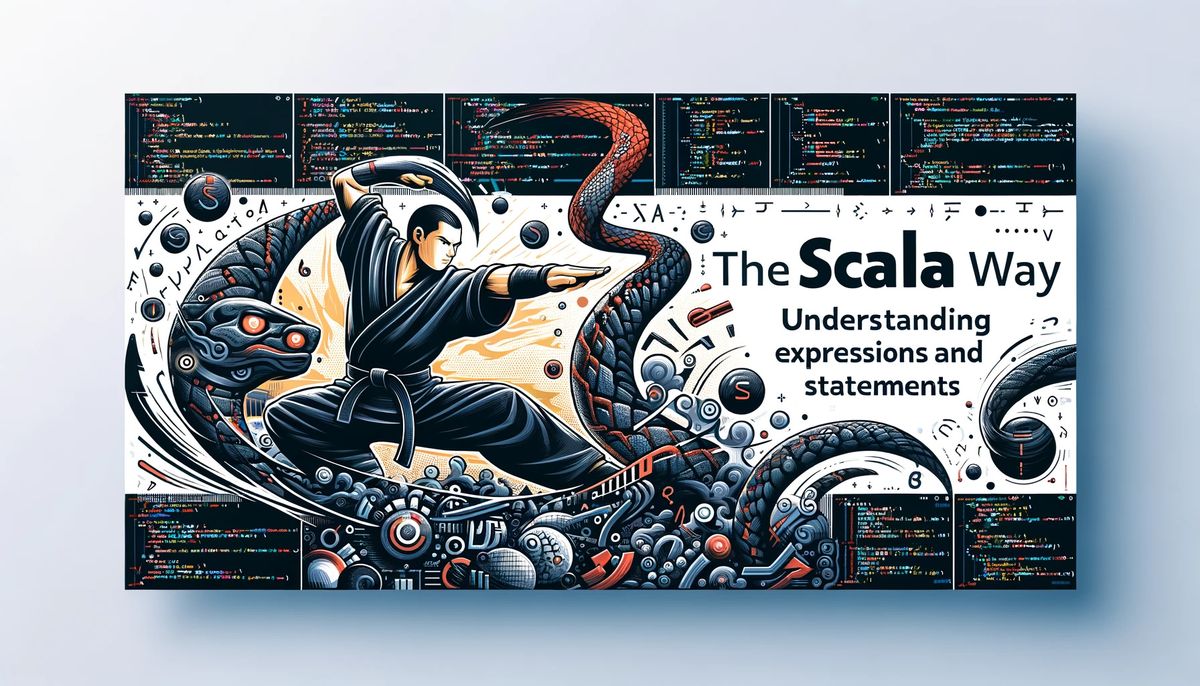
The distinction between expressions and statements is more than mere syntax; it's a window into the language's philosophy. Most programming languages have both, but functional languages will have an expression bias, while imperative ones will have many more statements.
With its functional programming roots, Scala leans heavily on expressions, transforming even traditionally statement-centric constructs into value-producing expressions. This shift affects the syntax and fundamentally changes the approach to coding, leading to more concise, expressive code.
Understanding this distinction is essential for anyone looking to master Scala.
The Scala Way: Understanding Expressions and Statements
Expressions are codes that can be evaluated to yield a value; hence, they can be used on the right side of an assignment. This includes operations like println
, which returns Unit
, a type representing a lack of a meaningful value but still considered a value in Scala:
val result = 3 + 4 // Expression
val unitExample = println("This returns Unit") // Expression returning Unit
// while loops are also expressions that always return Unit
val result = while (counter < 5) {
counter += 1
}
Scala's class and method declarations or imports are statements that do not produce a value and, hence, cannot appear on the right side of an equal sign.
As developers, we can use statements but can't write new ones. They are integral to Scala's structure and are defined by the language's design and compiler:
- Class declarations (
class MyClass {...}
) - Method definitions (
def myMethod(): Unit = {...}
) - Imports (
import scala.collection.mutable.ArrayBuffer
)
For instance, the following code will result in compilation errors:
val myClass = class MyClass {}
// Error: expression expected but class found
val myFunction = def myMethod(): Unit = {}
// Error: expression expected but def found
val myPackage = package test
// Error: expression expected but package found
Elvis Is Not in the Building
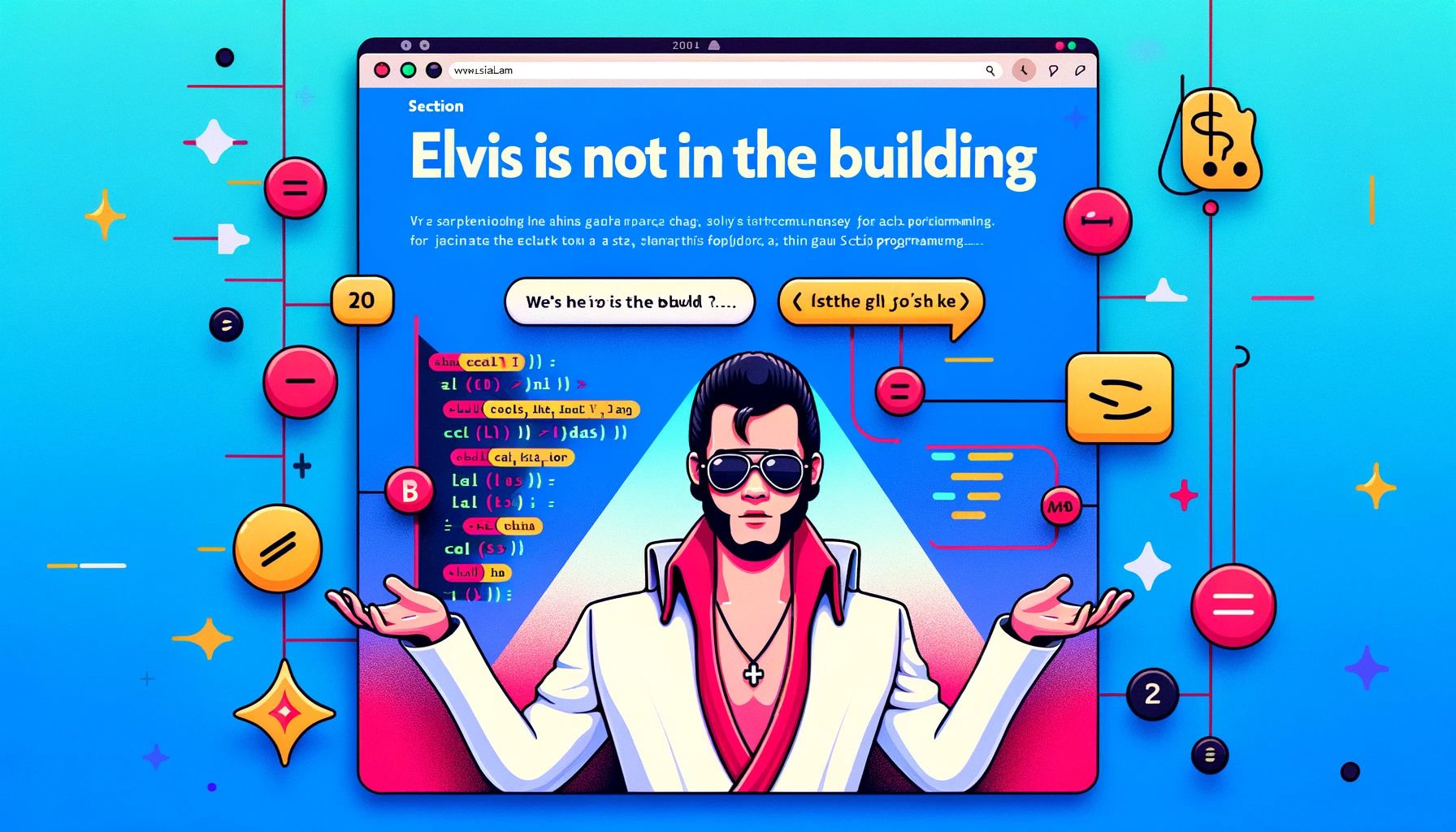
In Scala, the absence of the ternary operator (often jokingly referred to as the "Elvis operator" in other languages) is a direct consequence of its design philosophy.
Unlike many statement-oriented languages, Scala treats if
as an expression, not a statement. This means that if
in Scala inherently returns a value, similar to the ternary operator in languages like Java.
For example, in Scala, you can write:
val result = if (condition) value1 else value2
This eliminates the need for a separate ternary operator (condition ? value1 : value2
), streamlining the language's syntax and reinforcing its commitment to expression-oriented programming.
This shows how choosing to make the language expression-oriented simplified the syntax and overall complexity of the language, a core aspect of functional programming.
Conclusion
In summary, Scala's distinct handling of expressions and statements enhances the language's flexibility and readability and simplifies it.
This simplification is evident in Scala's if
expression, making it unnecessary to include an extra conditional operator like Java or Groovy.
Grasping these concepts allows developers to write more streamlined and expressive code. As we delve deeper into Scala's features, the language's capacity for simplifying complex programming paradigms will become increasingly clear, reinforcing Scala's position as a robust and efficient tool for programmers.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
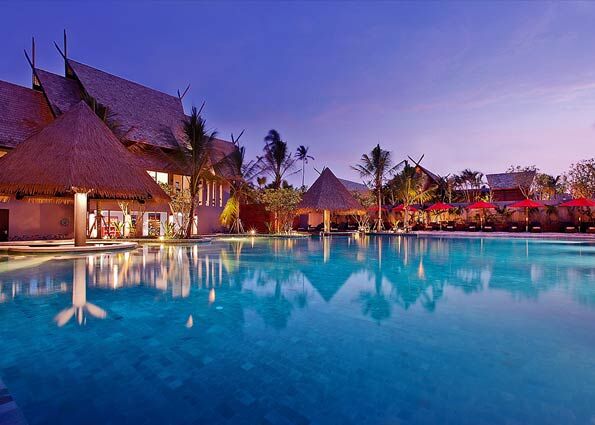
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: