Learn Conditional Statements in Python With Turtle
Dive into the basics of conditional logic with Python's Turtle module in our latest guide. Perfect for beginners, this article simplifies the essentials of using if-else statements to control the flow of your Python programs, complete with interactive examples.
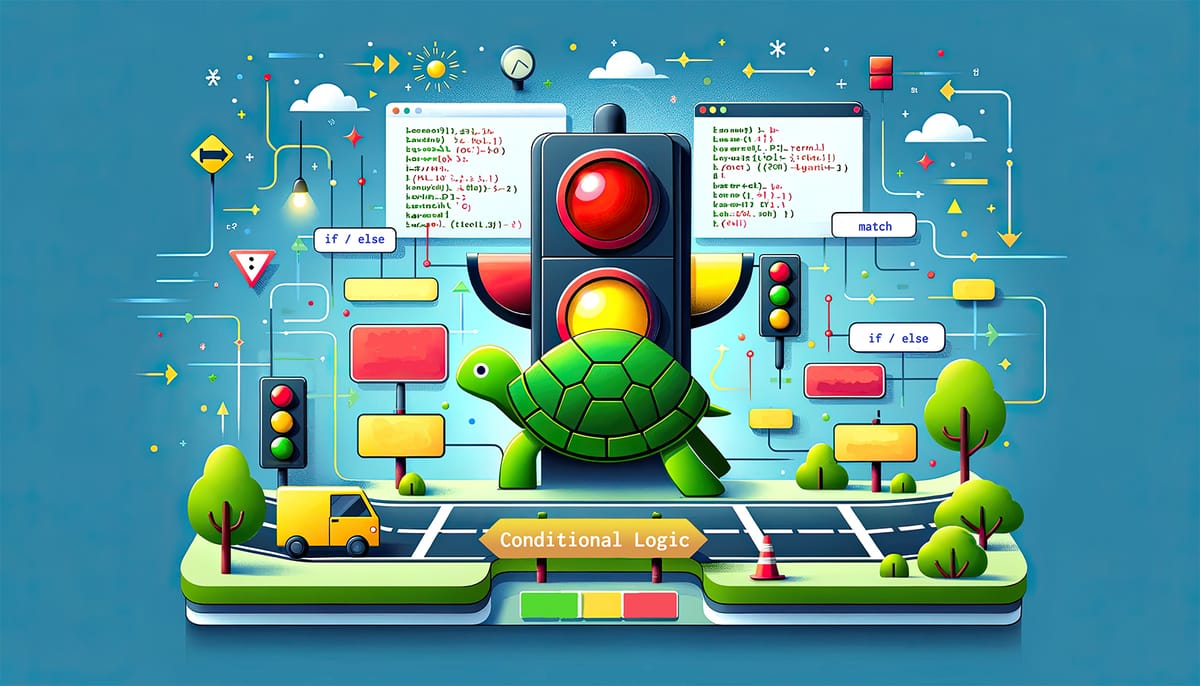
Overview
Welcome back to another exciting chapter in your Python programming adventure! Building on our previous exploration of loops with Python's Turtle module (as seen in "Creative Coding: An Introduction to Loops in Python with Turtle"), we now focus on the foundational concept of conditional logic.
In this article, we'll learn how to make decisions in your code using if-else statements. Our journey will take a creative turn, employing the charming Turtle module to demonstrate these concepts visually.
Additionally, we'll venture into the territory of pattern matching, a more recent feature in Python, providing an intriguing comparison to traditional conditional structures. Let's continue our coding journey with a fun, interactive project that brings these concepts to life!
Understanding Conditional Statements in Python
Conditional statements are at the heart of decision-making in Python. They allow our program to react differently to varying conditions, choosing different execution paths based on specific criteria.
In this section, we'll explore the foundational conditional statements in Python: if
, else
, and elif
.
Understanding if
Statements
The if
statement is the most basic form of conditional logic in Python. It evaluates a condition, and if it is True
, it executes a code block. The syntax is straightforward:
temperature = 75
if temperature > 70:
print("It's a warm day.")
In this case, if temperature
is greater than 70, the program prints "It's a warm day.".
Nothing will be printed if we change the value to 65, and the program will terminate. In summary, Python will execute the code below the if
only when the condition is true.
Learn Conditional Logic in Python With Turtle
Often, you'll want your program to do something when the if
condition isn't met. This is where else
comes into play. An else
statement follows an if
statement and contains code that executes when the if
condition is False
:
if temperature > 70:
print("It's a warm day.")
else:
print("It's not very warm today.")
If the temperature
is 70 or below, the program will print: "It's not very warm today."
Using elif
for Multiple Conditions
Sometimes, you'll encounter situations where you need to check multiple conditions. elif
, short for "else if", is used for this purpose. We can have as many elif
statements as necessary to check various conditions in sequence:
if temperature > 80:
print("It's hot outside.")
elif temperature > 60:
print("It's a nice day.")
else:
print("It might be a bit chilly.")
This structure allows for complex decision-making processes in our code.
A Visual Example With Turtle
Now that we've grasped the basics of conditional statements, let's see them in action with a fun and interactive example using Python's Turtle graphics. In this section, we'll create a simple traffic light simulation. In this example, we will illustrate conditional statements and visually represent how they guide program flow.
Setting Up the Traffic Light Simulation
First, let's set up our Turtle environment. We'll create a screen and a turtle that we'll use to represent the traffic light. Here's the initial setup:
import turtle
# Set up the screen
wn = turtle.Screen()
wn.title("Traffic Light Simulation")
wn.bgcolor("darkgrey")
# Create a turtle for drawing the traffic lights
light = turtle.Turtle()
light.shape("circle")
The update_light_color
Function
The core of our simulation is the update_light_color
function. This function uses conditional statements to change the color of our 'traffic light' based on the input it receives:
def update_light_color(color):
if color == "green":
light.color("green")
elif color == "yellow":
light.color("yellow")
elif color == "red":
light.color("red")
else:
print("Invalid color")
In this function, we use an if
statement followed by two elif
statements and an else
statement. The traffic light changes to green, yellow, or red depending on the input. If the input is anything other than these colors, it prints "Invalid color."
Interactive Control with Keyboard Events
To make our simulation interactive, we'll bind keyboard events to functions that change the traffic light's color. This is done using the onkey
method of the Turtle screen:
# Event handlers for keypresses to change the traffic light colors
def go_green():
update_light_color("green")
def slow_down():
update_light_color("yellow")
def stop():
update_light_color("red")
# Bind the keypresses to the event handlers
wn.onkey(go_green, "g")
wn.onkey(slow_down, "y")
wn.onkey(stop, "r")
# Listen to the keyboard events
wn.listen()
# Keep the window open until it's closed by the user
wn.mainloop()
In this setup, pressing 'g' on the keyboard triggers the go_green
function, changing the light to green and similarly for the other colors.
This example illustrates how conditional statements can be used to control the flow of a Python program. Integrating these concepts with Turtle graphics allows us to write functional code and see our logic in action.
Python's match
: A Modern Twist on Conditional Statements
Python 3.10 introduced a powerful feature known as the match
statement, an alternative to the traditional if-elif-else
chains commonly used for conditional logic. This feature, akin to the switch-case statements in other programming languages, offers a more concise and readable way to handle multiple conditional branches. Let's explore how using match
can improve our traffic light simulation.
Refactoring with match
In our previous traffic light example, we used a series of if
, elif
, and else
statements to determine the color of the traffic light. Here’s how we can refactor this using the match
statement:
def update_light_color(color):
match color:
case "green":
light.color("green")
case "yellow":
light.color("yellow")
case "red":
light.color("red")
case _:
print("Invalid color")
In this new structure, match
compares the variable color against several cases. If a case is matched, the corresponding block of code is executed.
Advantages of Using match
The match
statement comes with several benefits over the chained if-elif-else
approach:
- Readability and Clarity: The
match
statement clarifies that one checks a single variable against multiple potential values. This enhances readability, especially for those new to coding or others reading your code. - Conciseness: It reduces the boilerplate code often seen in lengthy
if-elif-else
chains. Withmatch
, each condition is neatly organized into cases, making the code more concise. - Maintenance: When modifying or extending our code,
match
makes it easier to understand and improve. Adding or changing cases is more straightforward, reducing the risk of errors when altering a complex chain ofif-elif-else
statements. - Pattern Matching Capabilities: Beyond simple value comparisons,
match
can be used for more advanced pattern matching, a powerful feature for more complex data structures and scenarios.
While the if-elif-else
chain is a staple in conditional logic, the match
statement in Python offers an elegant alternative, especially useful in scenarios with multiple conditions. By employing match
in our traffic light simulation, we achieved a more readable and maintainable code structure, demonstrating how Python continues to evolve with features that enhance the ease and efficiency of programming.
Conclusion: Embracing the Future of Python Programming
As we've journeyed through the realms of conditional logic in Python, from the foundational if
, else
, and elif
statements to the innovative match
statement, it's clear that Python continues to evolve, offering more elegant and efficient ways to write code.
The match
statement, a relatively new addition to Python's toolkit, represents a significant step forward in writing more readable and maintainable code. It's a testament to Python's ongoing commitment to developer productivity and code clarity. By incorporating these modern practices, you're keeping up with the latest programming trends and enhancing your problem-solving skills.
Please don't hesitate to modify the examples we've talked about, or better yet, create your unique projects.
In the ever-evolving world of programming, staying curious and adaptable is crucial. With its robust community and continuous updates, Python provides an excellent platform for lifelong learning and creativity in coding. So, keep exploring, keep coding, and most importantly, enjoy the process!
Happy coding!
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
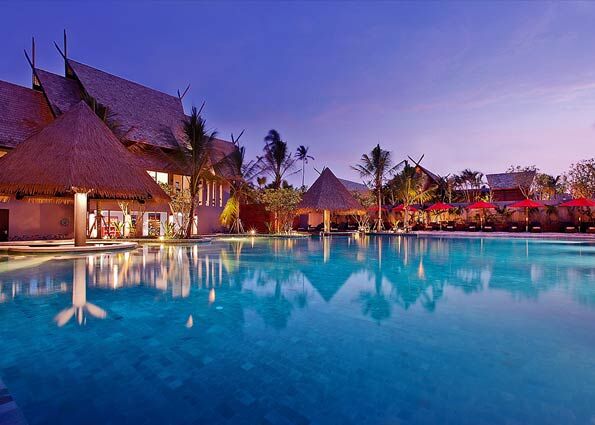
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: