Object-Oriented Python: A Turtle’s Guide to Classes
Explore Python's object-oriented side with 'A Turtle’s Guide to Classes.' Learn to structure code using classes and inheritance, illustrated through engaging Turtle graphics. Perfect for honing your Python skills in a fun, practical way!
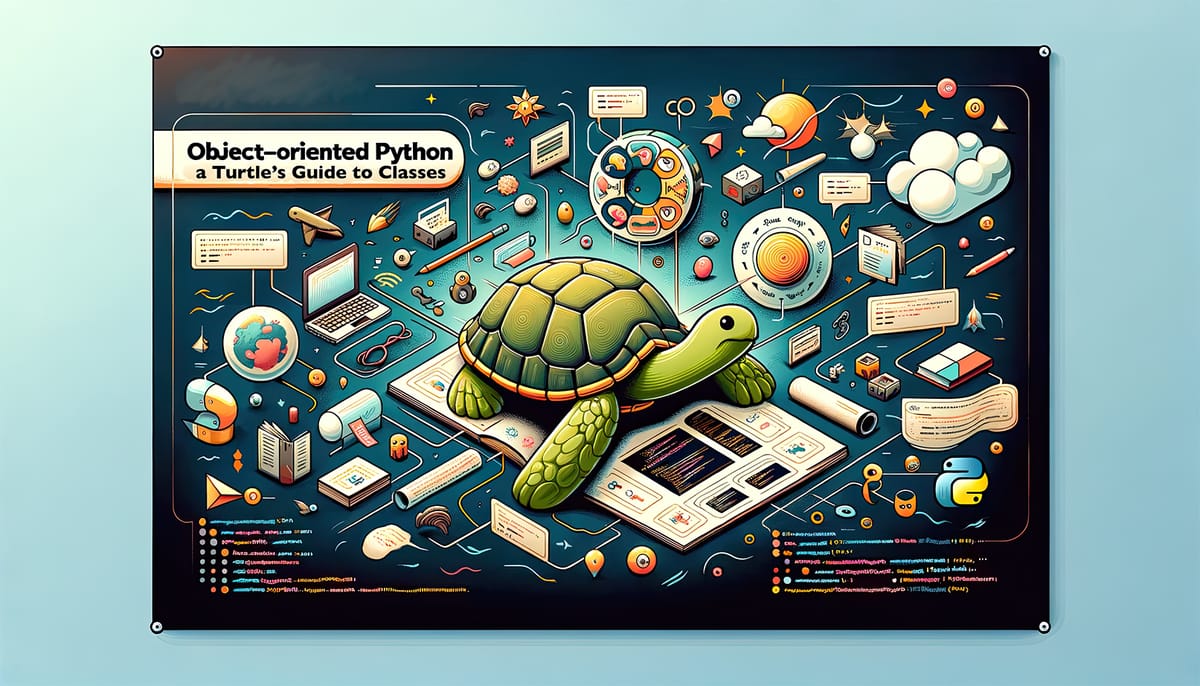
Introduction
In our previous adventures, we've delved into the fundamentals of Python, unraveling the mysteries of loops, branching, and functions. Now, we're ready to take a step further.
Welcome to "Object-Oriented Python: A Turtle’s Guide to Classes," where we embark on an exciting journey to explore the world of object-oriented programming (OOP) in Python through a turtle's eyes.
Object-oriented programming is a crucial aspect of Python, offering a structured approach to writing software. OOP is about organizing code resembling real-world entities and relationships, making our programs more intuitive to understand and easier to maintain.
In this article, we'll introduce you to Python classes, the backbone of OOP in Python. Using the familiar and friendly Turtle module, we'll demonstrate how classes can bring a new level of structure and clarity to your coding projects.
We'll start with defining and using classes, then gradually move to more complex concepts like inheritance and polymorphism.
So, let's dive in and discover the power and elegance of Python's object-oriented features, with our trusty Turtle leading the way!
From Functions to Classes: Understanding the Need for a More Structured Approach
In our journey through Python, we've learned how to use functions to encapsulate and execute specific tasks. While functions are an invaluable tool in programming, the need for a more structured and organized approach becomes evident as the complexity of projects increases.
This is where classes, a key concept in object-oriented programming (OOP), enter.
The Limitations of Functions in Complex Projects
Functions are excellent for performing defined operations but have limitations in handling complex systems.
For instance, consider a Python project that simulates an ecosystem. Using just functions, you'd have to manage numerous details like each animal's attributes and behaviors separately, leading to a tangled web of interdependent functions. This makes the code more challenging to manage and understand, increasing the risk of bugs.
Why Classes Offer a Better Solution
Classes provide a more elegant solution to these challenges. They allow us to create objects that encapsulate data and the functionality that operates on that data. This encapsulation makes understanding and managing complex systems in our code more manageable.
- Organized Data Management: With classes, related attributes are bundled together, making it clear which properties belong to which entity.
- Enhanced Code Reusability: Classes promote reusability by creating multiple instances of a class. Through inheritance, we can define new classes based on existing ones, inheriting their attributes and behaviors while adding new features.
- Simplified Maintenance: Changes in one part of a class do not affect other parts of your program as long as the interface remains consistent. This makes classes more straightforward to maintain and extend over time.
Basic Structure of a Python Class
Let's illustrate this with a basic example:
class Animal:
def __init__(self, species, habitat):
self.species = species
self.habitat = habitat
def describe(self):
print(f"The {self.species} lives in {self.habitat}.")
my_animal = Animal("Lion", "Savannah")
# Output: The Lion lives in Savannah.
my_animal.describe()
In summary, while functions are excellent for executing tasks, classes offer a more structured approach to representing and managing complex data and behaviors in code. They provide a foundation for organizing and scaling our Python projects, especially as we model more complex systems. In the following sections, we'll see how this concept applies beautifully to graphical programming with Turtle.
Understanding Python Classes through a Turtle Race
Now that we've introduced the concept of classes let's bring the idea to life by creating a Python class that interacts with the Turtle module.
Scenario: A Turtle Race
Imagine we're creating a simulation of a turtle race, where each turtle has its color, speed, and position.
Using functions alone would require managing these properties separately and passing them around, which can quickly become unwieldy. On the other hand, classes allow us to bundle each turtle's properties and behaviors, making the code more organized and manageable, and then use those classes to create multiple.
Creating a Turtle Racer Class
We'll define a TurtleRacer
class that encapsulates properties like color, speed, and position, along with methods to move the turtle:
import turtle
import random
class TurtleRacer:
def __init__(self, color, position):
self.turtle = turtle.Turtle(shape="turtle")
self.turtle.color(color)
self.turtle.penup()
self.turtle.goto(position)
def race(self):
distance = random.randint(1, 10)
self.turtle.forward(distance)
In this class:
__init__
sets up each turtle with a given color and starting position.race
is a method that moves the turtle forward by a random distance, simulating the race.
Managing Multiple Racers
Let's create multiple instances of TurtleRacer
and see how classes make it easier to manage each racer:
# Set up the race
racers = [
TurtleRacer("red", (-100, 20)),
TurtleRacer("blue", (-100, -20)),
TurtleRacer("green", (-100, -60))
]
# Start the race
for _ in range(10):
for racer in racers:
racer.race()
turtle.done()
In this example, each instance of TurtleRacer
maintains its state (color and position) and behavior (racing forward). The advantages here are:
- Encapsulation: Each racer's properties and actions are bundled within a single entity (the object). This makes the code cleaner and easier to understand.
- Code Reusability: The
TurtleRacer
class can be instantiated as often as needed without redundancy. - Scalability: Adding more racers or introducing new properties and methods to the
TurtleRacer
class is straightforward, demonstrating how classes support scalability.
This turtle race example demonstrates how classes offer significant advantages over standalone functions, particularly in scenarios involving multiple objects with distinct properties and behaviors.
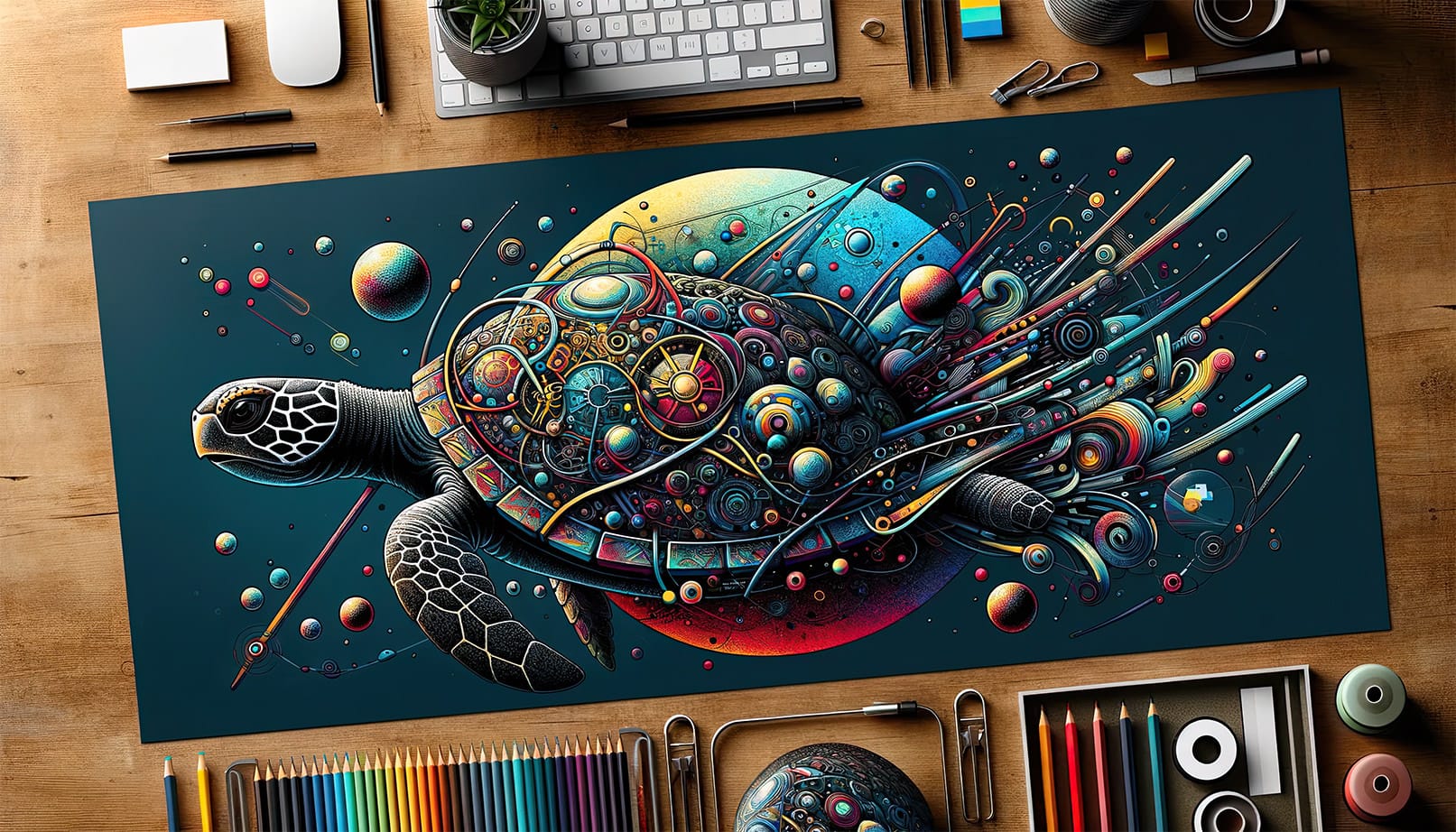
Introducing Inheritance: Building on Existing Classes
One of the most powerful features of object-oriented programming in Python is inheritance — the ability to create new classes that extend the functionality of existing ones. Inheritance promotes code reuse and a hierarchical organization of classes, making it easier to understand and maintain.
What is Inheritance?
Inheritance allows a new class (a child or subclass) to inherit attributes and methods from an existing class (the parent or superclass). This means the subclass can use the same functionality as the parent class and have unique features.
Basic Example of Inheritance
Let's extend our TurtleRacer
class from the previous example to demonstrate inheritance:
class FastTurtleRacer(TurtleRacer):
def __init__(self, color, position, speed):
super().__init__(color, position)
self.speed = speed
def turbo_boost(self):
self.speed = self.speed + 5
def race(self):
self.turtle.pendown()
self.turtle.forward(self.speed)
In this FastTurtleRacer
class:
- We inherit from
TurtleRacer
. - The
super().__init__(color, position)
call initializes the parent class. - We add a new attribute
speed
and a new method,turbo_boost
, to increase the speed. - And finally, we wrote a new version of the race method to use the speed to move the turtle at the given speed.
Utilizing the Subclass
Now, let's create instances of FastTurtleRacer
and see inheritance in action:
# Creating an enhanced racer
turbo_racer = FastTurtleRacer("purple", (-100, 60), 3)
turbo_racer.turbo_boost()
# Set up the race
racers = [
TurtleRacer("red", (-100, 20)),
TurtleRacer("blue", (-100, -20)),
TurtleRacer("green", (-100, -60)),
turbo_racer
]
# Start the race
for _ in range(50):
for racer in racers:
racer.race()
turtle.done()
In the race, the custom turbo_racer.race()
method is called during the race without changing the race code. We create an instance of the enhanced class and pass it to the same race code.
Advantages of Inheritance
Inheritance has several critical benefits in object-oriented programming:
- Reusability: It allows us to reuse code from parent classes, reducing redundancy.
- Extendibility: We can extend or modify the behavior of parent classes in a structured way.
- Hierarchy: It creates a natural hierarchy in code, making it easier to trace and understand the relationships between different classes.
Inheritance in Python classes provides a robust mechanism for building upon existing code, allowing us to extend functionality in an organized manner. We can create more complex and versatile classes with minimal code duplication by applying inheritance, as shown in our Turtle racing example.
This concept is fundamental in Python and forms the basis for more advanced object-oriented programming techniques.
Best Practices for Effective Class Design
Designing classes effectively is an art that improves with practice and understanding of key principles. Here are some best practices to keep in mind when designing classes in Python, especially when using them in conjunction with modules like Turtle for graphical programming.
1. Keep It Simple and Intuitive
- Single Responsibility Principle: Each class should have one primary responsibility and not be overloaded with multiple functionalities. This makes the class easier to understand and maintain.
- Avoid Over-Engineering: Start with a simple design and only add complexity when it's necessary. Overly complex classes can become difficult to maintain and understand.
2. Use Descriptive Names
- Class Names: Use CapWords convention (e.g.,
TurtleRacer
). Class names should be nouns and clearly represent what the class is about. - Method Names: Use lowercase with words separated by underscores (e.g.,
draw_circle
). Method names should be verbs indicating what the method does.
3. Encapsulate Internal Details
- Limit Direct Access to Attributes: Use private (prefixed with
_
) or protected (prefixed with__
) access modifiers to hide the object's internal state. This encapsulation protects the object's integrity and prevents unintended side effects. - Provide Public Methods for Interaction: Allow interaction with the object’s state through public methods. This provides a controlled interface for the object's functionality.
4. Ensure Reusability and Extensibility
- Design for Extensibility: Build classes that can be easily extended or composed with other classes. This encourages code reuse and flexibility in your design.
- Prefer Composition over Inheritance: Inheritance is a powerful tool but introduces potential maintenance challenges. For this reason, it is better to favor composition over inheritance.
- Make Use of Class and Static Methods: Understand when to use
@classmethod
and@staticmethod
to provide functionality that belongs to the class and is not necessarily an instance.
5. Write Readable and Maintainable Code
- Document Classes and Methods: Use docstrings to explain the purpose and usage of your classes and methods. This is invaluable for anyone who needs to read or modify your code later.
- Follow Python Coding Standards: Adhere to PEP 8 style guidelines to make your code more readable and consistent with the broader Python community.
Effective class design is crucial for creating robust and scalable Python applications. By following these best practices, your classes will serve their intended purpose effectively and integrate well with larger codebases and projects. Whether drawing simple shapes with Turtle or building complex software systems, these principles will guide you toward writing better, more efficient object-oriented code.
Conclusion
As we conclude our exploration of Python classes with a touch of Turtle graphics, we've journeyed through the foundational aspects of object-oriented programming, witnessed the elegance of classes in organizing code, and embraced the power of inheritance to extend functionality.
This adventure into Python's object-oriented features has equipped us with the tools to structure our code more effectively and opened up a world of creative possibilities.
The beauty of classes lies in their versatility and power to model real-world scenarios, making our code more intuitive and scalable. We encourage you to experiment with these concepts as you continue your Python journey. Try creating new classes, play with inheritance, or even integrate classes with other Python features you've learned.
Remember, learning Python is a continuous journey. Each concept you master opens new doors and possibilities. Whether you're creating simple Turtle drawings or building complex software systems, the principles of object-oriented programming will serve as a valuable guide.
Addendum: A Special Note for Our Readers
Before we sign off, we’d like to share something special with our readers who’ve appreciated the visual journey accompanying our articles.
We invite you to visit the TuringTacoTales Store on Redbubble. Here, you can explore a range of products featuring these unique and inspiring designs.
Each item showcases the beauty and creativity inherent in coding. It’s a perfect way to express your love for programming while enjoying high-quality, artistically crafted merchandise.
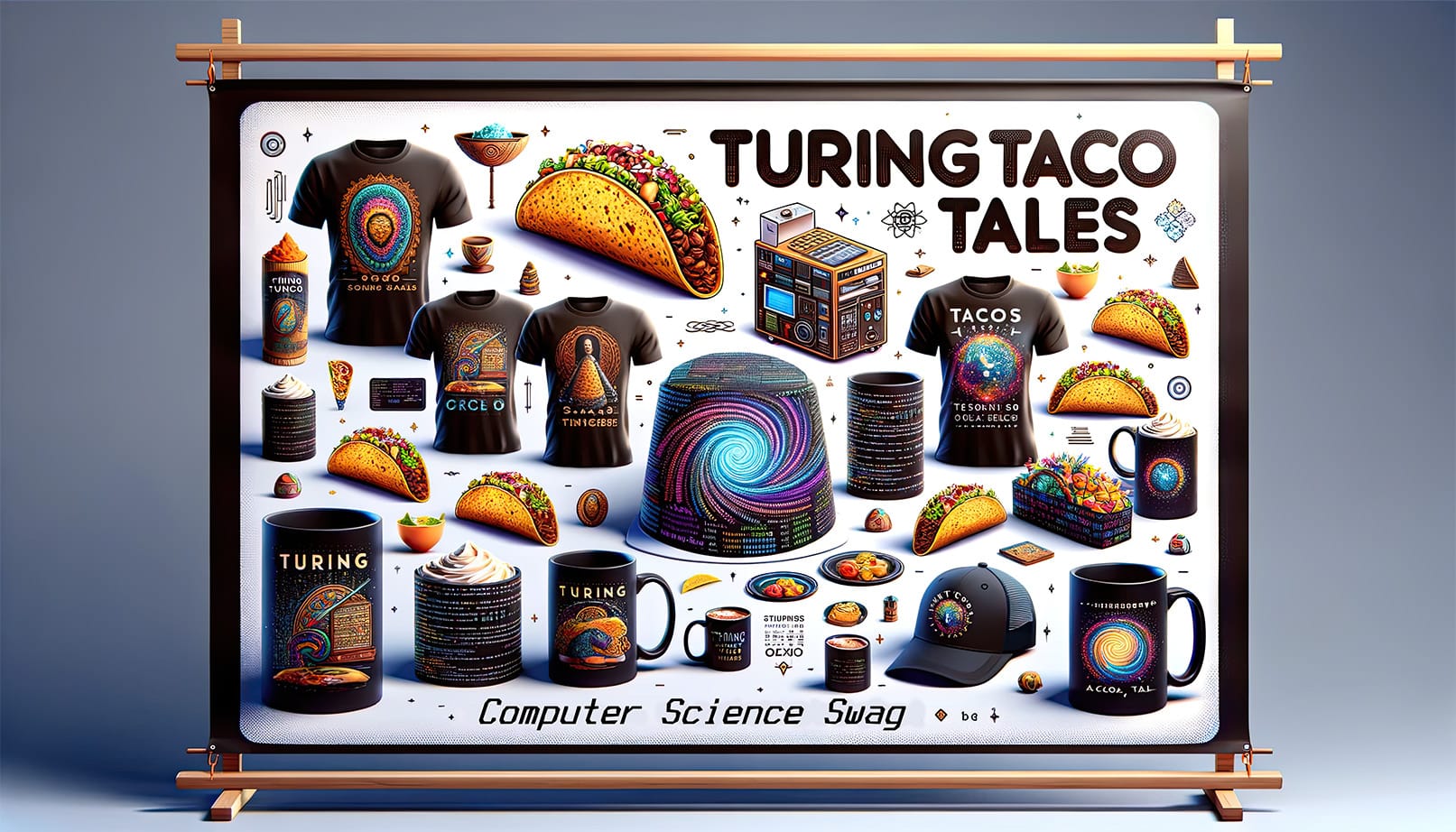
So, please take a moment to browse our collection and perhaps find something that resonates with your passion for Python and programming!