The Art of Python Functions: Designing Modular Masterpieces
TuringTaco.com's article on Python functions discusses their importance in modular coding. It covers the basics and practical uses with Turtle graphics and ends with a complex mandala pattern, showcasing the functions' role in organized, efficient coding.
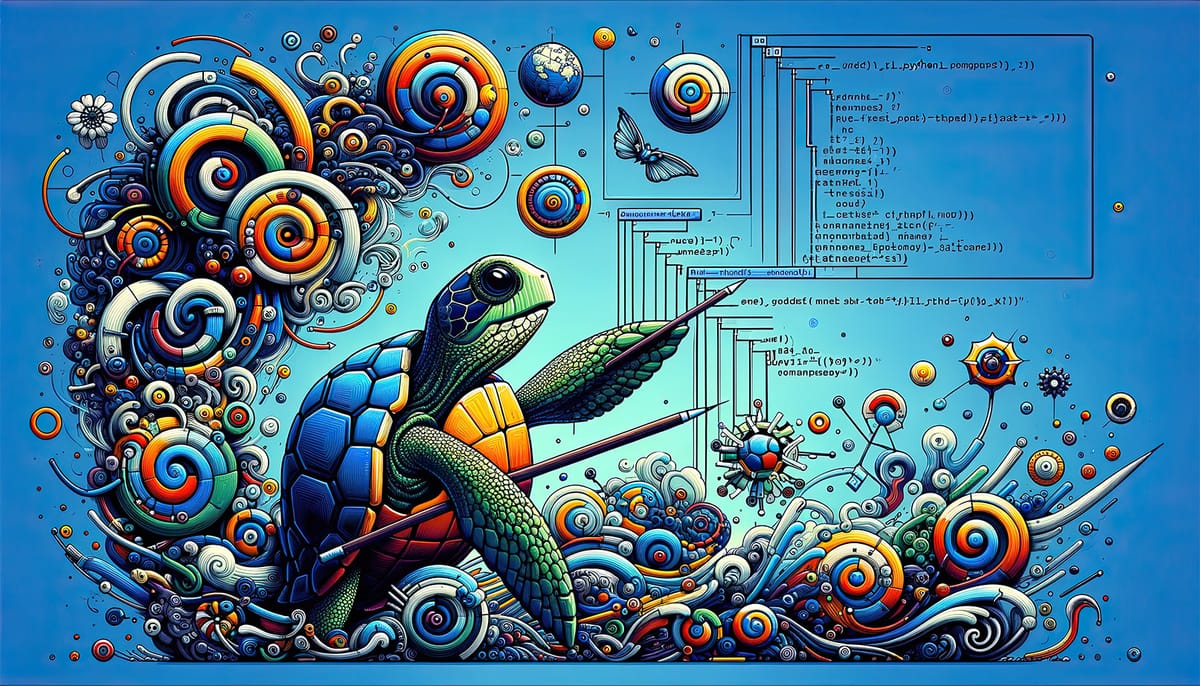
Overview
Welcome back to our ongoing exploration of Python's captivating world, where coding meets creativity!
We started our journey with Python's loops in "Creative Coding: An Introduction to Loops in Python with Turtle" and delved into the intricacies of branching in "Learn Statements Logic in Python with Turtle". This time, we're diving into Python functions and modularity, a key programming element.
Functions in Python are like magical spells in a programmer’s grimoire. They encapsulate specific tasks into reusable code blocks, which we can invoke whenever necessary.
In this article, we’ll create functions for shapes that we’ll reuse in different places in our code, mesmerizing shapes and patterns using Python’s Turtle graphics.
Understanding Python Functions
All programming languages have essential building blocks, some of which are repeat code and conditional execution. Yet, one of the most important ones is to avoid repeating code by creating callable blocks; every programming language has a feature for it.
Some focus on organizing and reusing big chunks of code like Classes, Objects or modules. But also, every language has a feature to reuse code in the small like subroutines, procedures, or the most commonly used name in later years: Functions.
What are Functions?
In Python, a function is a named block of code designed to perform a specific task. Think of a function as a mini-program within your program. It can perform an operation, return a result, or modify specific data. Functions help in breaking down complex tasks into smaller, manageable parts. This simplifies your coding process and aids in troubleshooting and debugging.
Basic Syntax of Python Functions
The anatomy of a Python function is simple. It is defined using the def
keyword, followed by a name, parentheses, and a colon. Inside the parentheses, we can specify parameters, which are inputs that the function can accept to perform its task.
Here's a basic template:
def function_name(parameters):
# Code to execute
return result # optional
Why Use Functions?
There are several compelling reasons to use functions in your coding endeavors:
- Reusability: Once defined, a function can be used multiple times throughout our program. This means we can write the code once and use it as many times as needed, avoiding redundancy.
- Modularity: Functions allow us to segment the code into separate distinct sections. We can develop, test, and debug each function independently, making our program more manageable.
- Clarity and Organization: Well-named functions can make our code much more readable. When we come back to read or modify it after some time, or when someone else reads our code, functions can provide clear insights into what each segment of your code should do.
- Scope Management: Functions help manage the scope of variables used within them. Variables defined inside a function are not accessible outside of it, preventing potential conflicts in the program.
As we progress in this article, we'll see these benefits, especially in drawing shapes with the Turtle module.
Drawing with Code: Mastering Functions in Python for Creative Designs
With the basics of Python functions under our belt, let's put this knowledge into practice. As in previous articles, we'll start by using the Turtle module to create a function that draws a shape. This hands-on approach will reinforce the concepts of functions and showcase the beauty of coding as an artistic tool.
Creating a Simple Shape-Drawing Function
Let's begin by defining a function to draw a square. This example will demonstrate how a simple function is structured and used in Python:
import turtle
# Function to draw a square
def draw_square():
turtle.begin_fill()
for _ in range(4):
turtle.forward(100) # Move turtle forward by 100 units
turtle.right(90) # Rotate turtle by 90 degrees
turtle.end_fill()
# Setting up the turtle environment
screen = turtle.Screen()
screen.title("Python Functions with Turtle")
# Draw the square
draw_square()
# Keep the window open
screen.mainloop()
In this function, draw_square
, we use a loop to repeat the action of moving the turtle forward and turning it right, creating a square. The function encapsulates all the steps needed to draw a square, making it easy to repeat this action multiple times without rewriting the code.
Expanding the Function with Parameters
To enhance the flexibility of our draw_square
function, we can introduce parameters. Parameters allow us to pass values to a function, making it more versatile. Let's modify draw_square
to accept the size of the square as a parameter:
# Function to draw a square with a given size
def draw_square(size):
turtle.begin_fill()
for _ in range(4):
turtle.forward(size) # Size of the square
turtle.right(90)
turtle.end_fill()
# Draw squares of different sizes and positions
draw_square(50, (-150, -150))
draw_square(75, (-75, -75))
draw_square(150, (25, 25))
turtle.done()
Now, the draw_square
function can draw squares of any size, as specified when calling the function:
The Program Running and paints squares on the screen
Unleashing Creativity with Functions
The true power of functions in creative coding is realized when you combine them to create complex designs. You can create intricate and beautiful designs by defining different functions for different shapes or patterns and then calling them in various combinations.
In the upcoming sections, we'll explore more ways to use functions to build complex patterns and how these modular blocks of code can be arranged creatively.
Designing an Impressive Pattern with Functions
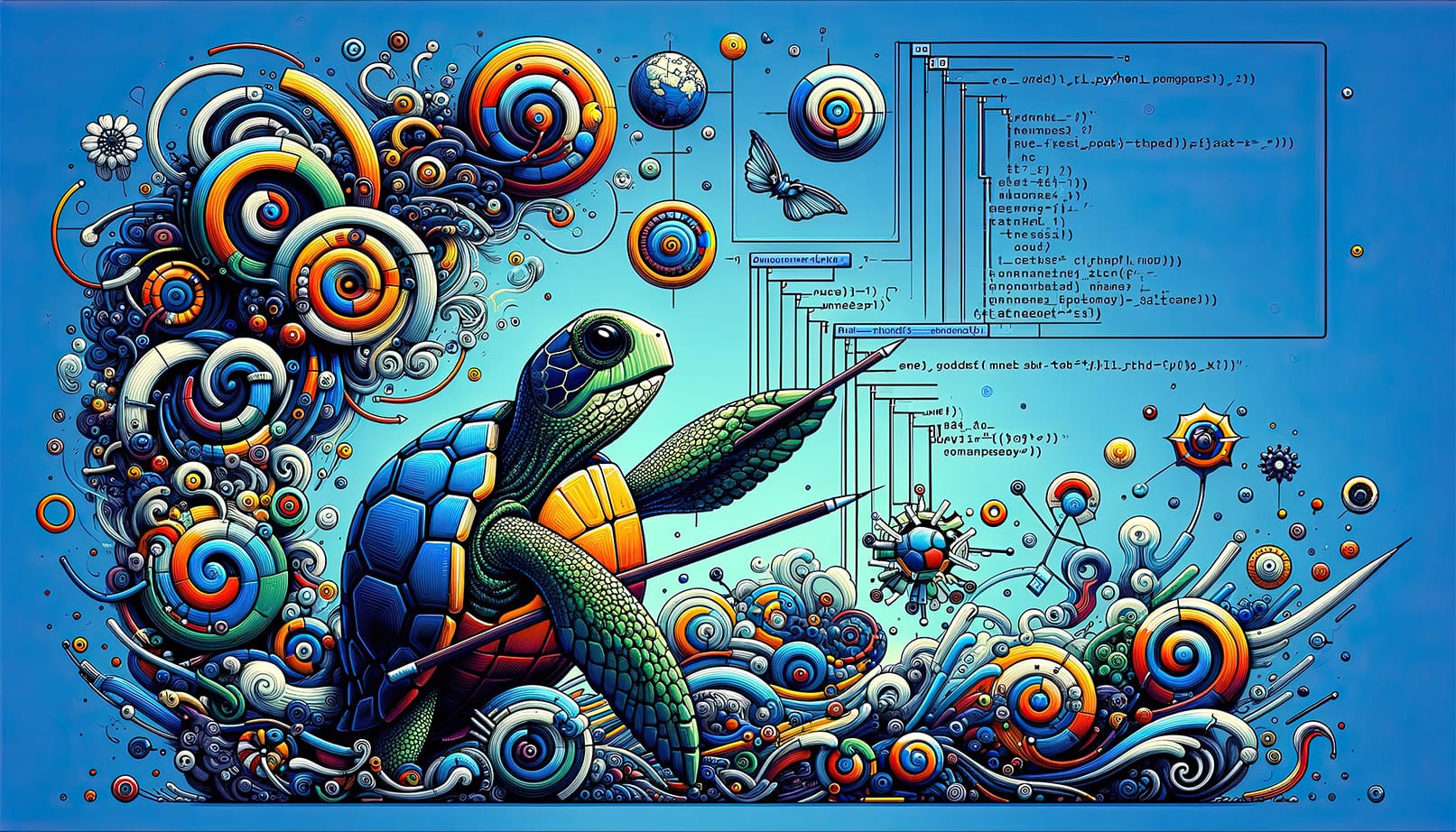
Now that we're familiar with using functions to draw individual shapes, let's stretch our creative muscles further. We'll design a more complex and visually striking pattern that beautifully illustrates the power of combining functions. In this example, we'll arrange multiple shapes in a circular, mandala-style pattern.
Creating a Function for the Mandala Pattern
First, let's define the functions of three basic shapes: triangle, square, and circle:
import turtle
def draw_square(size, position):
turtle.penup()
turtle.goto(position)
turtle.pendown()
for _ in range(4):
turtle.forward(size)
turtle.right(90)
def draw_circle(radius, position):
turtle.penup()
turtle.goto(position)
turtle.pendown()
turtle.circle(radius)
def draw_triangle(size, position):
turtle.penup()
turtle.goto(position)
turtle.pendown()
for _ in range(3):
turtle.forward(size)
turtle.right(120)
Next, let's define a function that draws a combination of shapes in a repeating pattern to form a mandala:
def draw_mandala(size, repeats):
for _ in range(repeats):
draw_square(size, turtle.position())
draw_circle(size / 2, turtle.position())
draw_triangle(size, turtle.position())
turtle.right(360 / repeats)
This function, draw_mandala
, takes two parameters: repeats
, which determines how many times the pattern repeats around the circle, and size
, which sets the size of the shapes.
Drawing the Mandala
Now, we'll use our draw_mandala
function to create the pattern. We can adjust the repeats
and size
parameters to change the appearance of the mandala:
turtle.speed('fastest') # Speed up the drawing
draw_mandala(200, 24) # Draw a mandala with 12 repeats and a size of 50
turtle.done()
The Result
This code creates a stunning mandala pattern composed of squares, circles, and triangles arranged symmetrically and circularly. The draw_mandala
function demonstrates how combining simple shapes with creativity can produce impressive results. The pattern's complexity and beauty are a testament to the power of functions in Python – allowing us to build sophisticated designs from basic building blocks.
We would like to encourage you to experiment with the draw_mandala
function. Try changing the number of repeats or the size of the shapes. Each adjustment will transform the pattern, unveiling new dimensions of its beauty. This exercise is a great way to practice coding with functions and an opportunity to witness the intersection of programming and art.
Conclusion
As we conclude our artistic journey with Python and Turtle, we've seen that functions are a powerful tool, not just a convenience. They allow us to make our code less repetitive, enable us to unleash our creativity, and help us write programs that would be intractable without them.
Key Takeaways
- Modularity and Reusability: Functions allow us to break down complex tasks into manageable parts, making our code more organized and reusable.
- Enhanced Readability: Well-defined functions make our code easier to read and understand, which is especially beneficial when returning to a project after some time or when sharing code with others.
The examples we've explored are just the beginning. The real adventure begins when you start experimenting with these concepts independently.
Try modifying the functions we've created or creating new ones to draw different shapes and patterns. Python's Turtle module provides a playful yet powerful canvas for your programming experiments.
If you've enjoyed this journey through the creative possibilities of Python and Turtle, don't let the adventure stop here! Subscribe to our newsletter to stay updated with more engaging and insightful content. By subscribing, you'll gain regular access to a treasure trove of Python tutorials, tips, and tricks designed to inspire and elevate your coding skills, no matter your level of expertise.
Addendum: A Special Note for Our Readers
I decided to delay the introduction of subscriptions. You can read the full story here.
If you find our content helpful, there are several ways you can support us:
- The easiest way is to share our articles and links page on social media; it is free and helps us greatly.
- If you want a great experience during the Chinese New Year, I am renting my timeshare in Phuket. A five-night stay in this resort in Phuket costs 11,582 € on Expedia. I am offering it in USD at an over 40% discount compared to that price. I received the Year of the Snake in style.
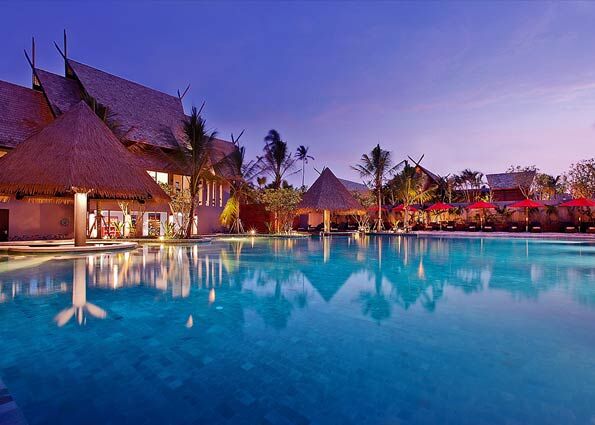
ReedWeek Timeshare Rental
- If your finances permit it, we are happy over any received donation. It helps us offset the site's running costs and an unexpected tax bill. Any amount is greatly appreciated:
- Finally, some articles have links to relevant goods and services; buying through them will not cost you more. And if you like programming swag, please visit the TuringTacoTales Store on Redbubble. Take a look. Maybe you can find something you like: